How do you write a save method for forms in django?
17,890
Solution 1
this could help you
def save(self):
data = self.cleaned_data
user = User(email=data['email'], first_name=data['first_name'],
last_name=data['last_name'], password1=data['password1'],
password2=data['password2'])
user.save()
userProfile = UserProfile(user=user,gender=data['genger'],
year=data['year'], location=data['location'])
userProfile.save()
Solution 2
The prefix
argument (also on ModelForm
by inheritance) to the constructor will allow you to put multiple forms within a single <form>
tag and distinguish between them on submission.
mf1 = ModelForm1(prefix="mf1")
mf2 = ModelForm2(prefix="mf2")
return render_to_response(..., {'modelform1': mf1, 'modelform2': mf2}, ...)
...
<form method="post">
{{ modelform1 }}
{{ modelform2 }}
...
</form>
Related videos on Youtube
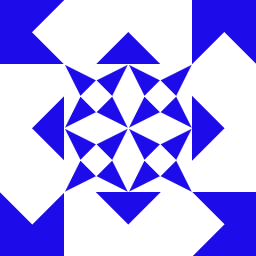
Author by
goelv
Updated on September 14, 2022Comments
-
goelv 3 months
I have two models in Django: User (pre-defined by Django) and UserProfile. The two are connected via a foreign key. I'm creating a form that allows a customer to edit their user profile. As such, this form will be based on both models mentioned.
How do I create a save() method for this form? What are the steps/requirements in completing the save function?
Here's what I have so far in forms.py:
class UserChangeForm(forms.Form): #fields corresponding to User Model email = forms.EmailField(required=True) first_name = forms.CharField(max_length = 30) last_name = forms.CharField(max_length = 30) password1 = forms.CharField(max_length=30, widget=forms.PasswordInput) password2 = forms.CharField(max_length=30, widget=forms.PasswordInput) #fields corresponding to UserProfile Model gender = forms.CharField(max_length = 30, widget=forms.Select) year = forms.CharField(max_length = 30, widget=forms.Select) location = forms.CharField(max_length = 30, widget=forms.Select) class Meta: fields = ("username", "email", "password1", "password2", "location", "gender", "year", "first_name", "last_name") def save(self): data = self.cleaned_data # What to do next over here?
Is this a good start or would anyone recommend changing this up before we start writing the save() function?
-
goelvCan I create a ModelForm that is based on two models? If so, how would I go about doing that?
-
-
goelv over 10 yearsJust to clarify / sake of learning, what is the purpose of saving the data as instances of the models? Is that what every form does, and if so why is that?
-
alexander over 10 yearsOnly based forms "ModelForm" have a built-save method. For forms based on "Form", you have to create a save method. ModelForms