Specifying include directories on the cmake command line
Solution 1
If the path to your headers is fixed in relation to your sources, then you should be able to avoid having to pass this info via the command line.
Say your project's directory structure is:
/CMakeLists.txt
/my_sources/main.cpp
/my_sources/foo.cpp
/my_includes/foo.hpp
and in your CMakeLists.txt, you currently have something like:
add_executable(MyExe my_sources/main.cpp my_sources/foo.cpp)
then to add the /my_includes
folder to the the list of include search paths, you only need to add the following:
include_directories(my_includes)
For further info about include_directories
, run
cmake --help-command include_directories
Answer to update in question:
Yes, using the -D
command line option just do
cmake . -DEO_SOURCE_DIR:PATH=<Path to required dir>
The variable ${EO_SOURCE_DIR}
gets cached as a result of this, so you only need this -D
argument once (unless the required path changes or you delete your CMakeCache file, etc.)
Solution 2
Proper way to do this is to define a variable in CMakeLists.txt and ask user to set it:
set(YOURLIB_INCLUDE_DIR "" CACHE FILEPATH "Path to yourlib includes")
if(NOT EXISTS ${YOURLIB_INCLUDE_DIR}/header.h)
message(SEND_ERROR "Can't find header.h in ${YOURLIB_INCLUDE_DIR})
endif()
include_directories(${YOURLIB_INCLUDE_DIR})
Now you can set it from the command line: cmake -D YOURLIB_INCLUDE_DIR=/path/to/yourlib/include .
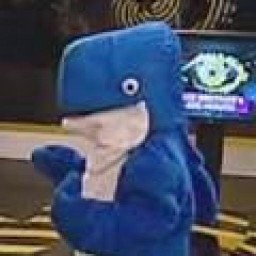
Olumide
Updated on October 09, 2020Comments
-
Olumide about 2 years
Is it possible to specify an include directory when running
cmake
. For examplecmake . -INCLUDE=/foo/bar
The header files are in a separate directory from the sources that I would like to compile, and I would like to remedy this without tinkering with the
Makefile
generated bycmake
.Update
The project does have a
CMakeLists.txt
. Excerpt:INCLUDE_DIRECTORIES(${EO_SOURCE_DIR}/src) INCLUDE_DIRECTORIES(${EO_SOURCE_DIR}/src/ga) INCLUDE_DIRECTORIES(${EO_SOURCE_DIR}/src/utils)
Can
${EO_SOURCE_DIR}
be set from the command line?-
tpg2114 about 10 yearsBut you can't modify the CMakeLists.txt?
-
Fraser about 10 years@Olumide See my updated answer.
-
-
Johannes about 9 yearsIs it possible to include multiple include directories from commandline with your solution?
-
arrowd about 9 yearsTry listing directories, separating them with
;
. -
Daniel Ziltener almost 5 yearsSure, but some devs are too stubborn to do this.