static_cast vs dynamic_cast
Solution 1
Use static_cast
. If you know that your Base*
points to a Derived
, then use static_cast
. dynamic_cast
is useful for when it might point to a derived.
Solution 2
When in doubt, you should prefer dynamic_cast
. It might be slower, but you probably won't notice the difference anyway.
If you need speed, use the following snippet:
template <typename Derived, typename Base>
Derived safe_static_cast(Base b)
{
assert((void*)dynamic_cast<Derived>(b) && "safe_static_cast failed!");
return static_cast<Derived>(b);
}
Or something equivalent.
The idea is that in debug builds, it checks that it's indeed what you thought it would be, and it release builds... well everything has already been tested, hasn't it ?
Solution 3
From MSDN -
In general you use static_cast when you want to convert numeric data types such as enums to ints or ints to floats, and you are certain of the data types involved in the conversion. static_cast conversions are not as safe as dynamic_cast conversions, because static_cast does no run-time type check, while dynamic_cast does. A dynamic_cast to an ambiguous pointer will fail, while a static_cast returns as if nothing were wrong; this can be dangerous. Although dynamic_cast conversions are safer, dynamic_cast only works on pointers or references, and the run-time type check is an overhead.
For more info, check out this link
Related videos on Youtube
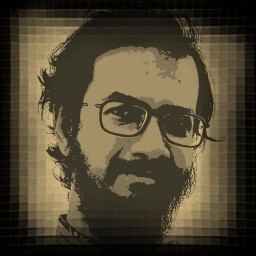
Comments
-
Donotalo 15 minutes
Suppose I'm given a C++ library full of inheritance. I'm given a
Base*
in a function when I know that it is actually pointing to aDerived
object andDerived
inheritsBase
. But I don't know what kind of inheritance it is (public/protected/private). I also don't know if there is any virtual function in the hierarchy.Given this situation, without looking into the source code/documentation of
Base
andDerived
, which cast should I use? Or should I consult the code/documentation first to ensure about polymorphism?Background
I am writing
changeEvent
function ofQMainWindow
in Qt 4.7. ThechangeEvent
function takesQEvent*
which I can cast to other type by knowingQEvent::type()
. I was wondering if I should usestatic_cast
ordynamic_cast
.Thanks.
-
Zac Howland over 11 yearsIf any library you use uses
protected
inheritance, it is time to find a better written library. -
Puppy over 11 years@Zac: Wish I could downvote comments. How can you possibly judge whether or not protected inheritance is the right tool for the job if you don't know what that library does?
-
-
Gene Bushuyev over 11 yearsyou don't need
(void*)
cast -
Matthieu M. over 11 years@Gene: it's admittedly ugly :) I was trying to avoid overloading for the reference case.
-
Martin York over 11 yearsYou might know now. But he is using a library and I am sure there is no gurantee provided by the library that they will not change implementation details. The interface is Base* so that is ALL you can assume. Therefore you MUST use dynamic_cast<> so that you can check that your assumptions hold afterwords.
-
Puppy over 11 years@Martin: That's his problem, not mine. If he knows that it does indeed point to a derived, then the root of that knowledge is out of the bounds of what I can possibly answer for.
-
Martin York over 11 yearsThe probelm is he can't know. His knowledge is limited to the time space cordinates of this one particular instance. Thus we need to emphasis that the OP is wrong in his assumption that he knows. He knows now but the knowledge is not transferable to the future. Thus because he is wrong static_cast is the wrong answer (it is the correct answer if the OP was correct(but he is not)). The only time you can assume the interface is Derived is when it returns a Derived* otherwise it may return anything derived from Base* (thats why we have interfaces in the code).
-
Puppy about 7 yearsJust for the record, I have used this and you can also use a typeid() check to check the types.