2 bytes to short java
Solution 1
Remember, you don't have to tie yourself in knots with bit shifting if you're not too familiar with the details. You can use a ByteBuffer to help you out:
ByteBuffer bb = ByteBuffer.allocate(2);
bb.order(ByteOrder.LITTLE_ENDIAN);
bb.put(firstByte);
bb.put(secondByte);
short shortVal = bb.getShort(0);
And vice versa, you can put a short, then pull out bytes.
By the way, bitwise operations automatically promote the operands to at least the width of an int. There's really no notion of "not being allowed to shift a byte more than 7 bits" and other rumours that seem to be going round.
Solution 2
When converting byte values from a stream into numeric values in Java you have to be very careful with sign extension. There is a trap with negative numbers (values from (unsigned) 128-255).
Try this (it works if hi and lo are any Java integer type) :
short val=(short)(((hi & 0xFF) << 8) | (lo & 0xFF));
I find it's best to be explicit with the parentheses in these cases.
Solution 3
This happens when trying to concatenate bytes (very subtle)
byte b1 = (byte) 0xAD;
byte b2 = (byte) 0xCA;
short s = (short) (b1<<8 | b2);
The above produces 0xFFCA, which is wrong. This is because b2 is negative (byte type is signed!), which means that when it will get converted to int type for the bit-wise | operation, it will be left-padded with 0xF!
Therefore, you must remember to mask-out the padded bytes so that they will definitely be zero:
short s = (short) (b1<<8 | b2 & 0xFF);
Solution 4
The other answers are OK, but I would like to put an emphasis on the type:
short high=(-48 & 0x00ff);
short low=80;
int c= ((high & 0xFF) << 8) | (low & 0xFF);
The short
type can represent values between -32768 to 32767. 53328 cannot be nicely stored in short, use int
instead as it allows you to store unsigned value up to ~109
So don't downcast the expression to short as it will net you the signed value.
Solution 5
You can convert 2 bytes to a short in a more readable and elegant way.
short s = ByteBuffer.wrap(new byte[]{0x01, 0x02}).getShort();
// now s equals 258 = 256 + 2
The first byte is the most significant byte.
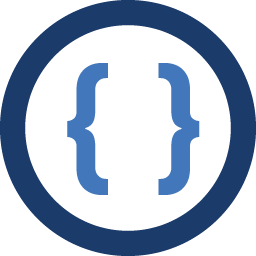
Admin
Updated on December 11, 2020Comments
-
Admin over 3 years
i'm reading 133 length packet from serialport,last 2 bytes contain CRC values,2 bytes value i've make single(short i think) using java. this what i have done,
short high=(-48 & 0x00ff); short low=80; short c=(short) ((high<<8)+low);
but i'm not getting correct result,is it problem because signed valued? how can i solve this problem,plz help me i'm in trouble