(-215:Assertion failed) !_src.empty() in function 'cv::cvtColor' with cv::imread
Solution 1
This means you are passing a Uninitialized variable to
> cv2.cvtColor()
After this statement:
# Read image with opencv
img = cv2.imread(img_path)
Can you try to print the img variable before passing to cv2.cvtColor() function
> print(img) or print(img.shape)
to make sure function call to read the image is successful
Solution 2
Error: !_src.empty() in function 'cv::cvtColor means the object passed to function cvtColor is empty or say none. Here, in the following line img is none
cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
and img is result of following function -
img = cv2.imread(img_path)
The possible reasons, why img can be empty object are -
- The image path is not correct, try absolute path and check image file extension.
- The image file is not accessible. There could be permission issue.
To avoid this error check if img object is None, if it is not none then only pass it to cvtColor function.
img = cv2.imread(img_path)
if(img is not None):
cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
Solution 3
This error occurs when the image is in one format and in the python program you specify other format.
Example:
File Path= /home/user/image.jpg
but in the python program you read the image as jpeg
img = cv.imread("image.jpeg")
Then you will be facing this error
Solution 4
If anyone came here searching this error message on Google but this error for you is for a video rather than an image, one possible reason might be you just ran out of frames to read from the video. reference
while True:
# Capture frame-by-frame
ret, frame = cap.read()
# if frame is read correctly ret is True
if not ret:
print("Can't receive frame (stream end?). Exiting ...")
break
# Our operations on the frame come here
gray = cv.cvtColor(frame, cv.COLOR_BGR2GRAY)
Using ret to check the end of the video is common practice but you may have forgotten to mention it or do the operations on the frame before checking if the frame is available or not (ret == True) like me. Cheers.
Solution 5
I think your source path should be:
src_path = "C:/Users/Benji's Beast/Desktop/"
Because in here get_string(src_path + "cont.jpg")
you've concatenated the image name.
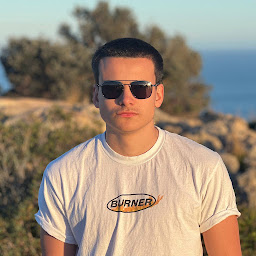
Benji
Updated on July 09, 2022Comments
-
Benji almost 2 years
I am trying to recognize text from an image to then have the text outputted; however, this error spits out:
Traceback (most recent call last): File "C:/Users/Benji's Beast/AppData/Local/Programs/Python/Python37-32/imageDet.py", line 41, in print(get_string(src_path + "cont.jpg") ) File "C:/Users/Benji's Beast/AppData/Local/Programs/Python/Python37-32/imageDet.py", line 15, in get_string img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) cv2.error: OpenCV(3.4.4) C:\projects\opencv-python\opencv\modules\imgproc\src\color.cpp:181: error: (-215:Assertion failed) !_src.empty() in function 'cv::cvtColor'
The image resolution is 1371x51. I have tried changing the "/" on src_path to "\" but that didn't work. Any ideas?
Here is my code:
import cv2 import numpy as np import pytesseract from PIL import Image from pytesseract import image_to_string # Path of working folder on Disk src_path = "C:/Users/Benji's Beast/Desktop/image.PNG" def get_string(img_path): # Read image with opencv img = cv2.imread(img_path) # Convert to gray img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Apply dilation and erosion to remove some noise kernel = np.ones((1, 1), np.uint8) img = cv2.dilate(img, kernel, iterations=1) img = cv2.erode(img, kernel, iterations=1) # Write image after removed noise cv2.imwrite(src_path + "removed_noise.png", img) # Apply threshold to get image with only black and white #img = cv2.adaptiveThreshold(img, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C, cv2.THRESH_BINARY, 31, 2) # Write the image after apply opencv to do some ... cv2.imwrite(src_path + "thres.png", img) # Recognize text with tesseract for python result = pytesseract.image_to_string(Image.open(src_path + "thres.png")) # Remove template file #os.remove(temp) return result print('--- Start recognize text from image ---') print(get_string(src_path + "cont.jpg") ) print("------ Done -------")
I have no idea how to fix this, thanks.