'Malformed UTF-8 characters, possibly incorrectly encoded' in Laravel
192,246
Solution 1
I wrote this method to handle UTF8 arrays and JSON problems. It works fine with array (simple and multidimensional).
/**
* Encode array from latin1 to utf8 recursively
* @param $dat
* @return array|string
*/
public static function convert_from_latin1_to_utf8_recursively($dat)
{
if (is_string($dat)) {
return utf8_encode($dat);
} elseif (is_array($dat)) {
$ret = [];
foreach ($dat as $i => $d) $ret[ $i ] = self::convert_from_latin1_to_utf8_recursively($d);
return $ret;
} elseif (is_object($dat)) {
foreach ($dat as $i => $d) $dat->$i = self::convert_from_latin1_to_utf8_recursively($d);
return $dat;
} else {
return $dat;
}
}
// Sample use
// Just pass your array or string and the UTF8 encode will be fixed
$data = convert_from_latin1_to_utf8_recursively($data);
Solution 2
I found the answer to this problem here
Just do
mb_convert_encoding($data['name'], 'UTF-8', 'UTF-8');
Solution 3
In my case I had a ucfirst
on the asian letters string. This was not possible and produced a non utf8 string.
Solution 4
In Laravel 7.x, this helped me to get rid of this error.
$newString = mb_convert_encoding($arr, "UTF-8", "auto");
return response()->json($newString);
Solution 5
I've experienced the same problem. The thing is that I forgot to start the apache and mysql in xampp... :S
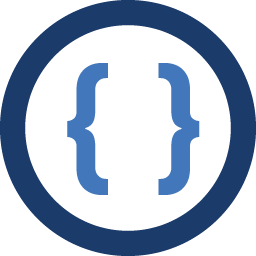
Author by
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
I'm using Laravel (a PHP framework) to write a service for mobile and have the data returned in
JSON
format. In the data result there are some fields encoded inUTF-8
.The following statement
return JsonResponse::create($data);
returns the error below
InvalidArgumentException HELP Malformed UTF-8 characters, possibly incorrectly encoded Open: /var/www/html/vendor/symfony/http-foundation/Symfony/Component/HttpFoundation/JsonResponse.php } catch (\Exception $exception) { restore_error_handler(); throw $exception; } if (JSON_ERROR_NONE !== json_last_error()) { throw new \InvalidArgumentException($this->transformJsonError()); }
I've changed:
return JsonResponse::create($data);
to
return JsonResponse::create($data, 200, array('Content-Type'=>'application/json; charset=utf-8' ));
but it still isn't working.
How can I fix it?