'numpy.float64' object has no attribute 'plot'
You've overwritten the pylab
module accidentally later on in your code by assigning something else to p
. You can avoid this by just importing pylab
and using, for example, pylab.plot
.
You've also got some indentation issues, remember that indentation matters in Python.
Using matplotlib.pyplot
is generally recommended as opposed to using pylab
. As such I've modified the code below to use pyplot
over pylab
. I've also removed some unneeded parts of the code and generally tidied it up.
import matplotlib.pyplot as plt
import numpy as np
from random import random
X=np.arange(0,1000)
y=np.random.randint(100,size=1000)
if len(X)==len(y):
print("ok")
else:
print("not ok")
polyfit=np.polyfit(X,y,6)
poly1d=np.poly1d(polyfit)
my=[]
for i in X:
p=poly1d(i)
my.append(p)
plt.plot(X,my)
plt.show()
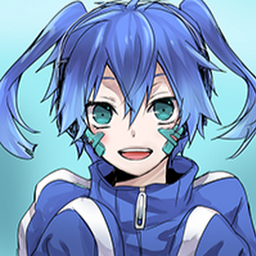
wiedzminYo
Updated on June 04, 2022Comments
-
wiedzminYo almost 2 years
I have a very simple code but at the end i found problem which I couldn't solve or find any solution. I can't draw plot. All I get is error AttributeError: 'numpy.float64' object has no attribute 'plot'
import pylab as p import numpy as np import sympy as s import matplotlib from random import random X=np.arange(0,1000) y=np.random.randint(100,size=1000) if len(X)==len(y): print "ok" else: print "not ok" polyfit=np.polyfit(X,y,6) poly1d=np.poly1d(polyfit) print poly1d i=1 my=[] for i in X: p=poly1d(i) my.append(p) print my p.plot(X,my) p.show()
I look after docs but I found nothing,google also can't help me.