'String' is not a subtype of type 'bool'
Solution 1
bool
are not supported in SQLite.
What is likely happening is that the isDone
column value true
is converted to the string "true"
so it is crashing in your TodoItem.map
constructor.
Try to convert (and parse) the value for example to 1 or 0 (int) before insertion or in query args.
Solution 2
This error appear on Flutter when bool x = string value assigned in the code. Unfortunately, there is no conversion from string to bool in Dart. One simple trick is to put something like this
String test = "true";
bool isCorrect = test == 'true';
This works as expected because the code will compare value of string and bool as strings and return the result as bool. You may need to use .toLowerCase() or .toUpperCase() according to your string value before the second line of the code above.
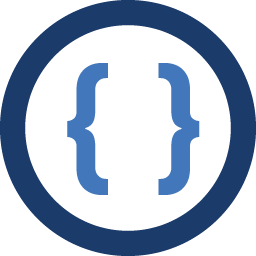
Admin
Updated on December 13, 2022Comments
-
Admin over 1 year
This is a todo list app which uses a database. I am using a boolean variable
isDone
to mark a task as complete but when i add a new task, i get the error'String' is not a subtype of type 'bool'
. I want to change the value ofisDone
to string but i am unsure on where exactly i can type the.toString()
line.Tried using the solution for similar errors (int, bool, future is not a subtype of string, bool, etc.) but could'nt solve it because those errors were application specific.
database code :
//This is the database String _itemName; String _dateCreated; int _id; bool _isDone; TodoItem(this._itemName, this._dateCreated, this._isDone); TodoItem.map(dynamic obj) { this._itemName = obj["itemName"]; this._dateCreated = obj["dateCreated"]; this._id = obj["id"]; this._isDone = obj["isDone"]; } String get itemName => _itemName; String get dateCreated => _dateCreated; int get id => _id; bool get isDone => _isDone; Map<String, dynamic> toMap() { var map = new Map<String, dynamic>(); map["itemName"] = _itemName; map["dateCreated"] = _dateCreated; map["isDone"] = _isDone; if (_id != null) { map["id"] = _id; } return map; } TodoItem.fromMap(Map<String, dynamic> map) { this._itemName = map["itemName"]; this._dateCreated = map["dateCreated"]; this._id = map["id"]; this._isDone = map["isDone"]; }
database create, update functions :
NOTE: the update function is only supposed to change the value of isDone
Future<bool> updateItem(TodoItem item) async { var dbClient = await db; int res = await dbClient.update("id", item.toMap(), where: "id = ?", whereArgs: <bool>[item.isDone]); return res > 0 ? true : false; }
Future<int> saveItem(TodoItem item) async { var dbClient = await db; int res = await dbClient.insert("$tableName", item.toMap()); print(res.toString()); return res; }
-
Amit Prajapati over 4 yearsCan you post that json as well, might ne map["isDone"] is coming in string for e.x "true"
-
Shagun Jain over 4 yearssqflite doesn't support boolean.It stores boolean value as 1 or 0 (i.e in the form of int) .Try using <int> instead of <bool>.Please let me know if it works or not.
-