'this' argument to member function 'select' has type 'const SelectParam', but function is not marked const
Solution 1
Indeed, you cannot call a non-const
method a const
object. But you also cannot call a non-const
method through a pointer or reference to a const
object (regardless of whether the referred-to object is const
or not).
This means that this:
const SelectParam* ptr = whatever();
ptr->select(someTuple);
is ill-formed.
In your case, you've declared a pointer to a const SelectParam
here on this line:
for (const SelectParam* p: selectionParams) {
Simply remove the const
and it should work :-)
On the other hand, if select
is never meant to modify the object, simply mark it as const:
virtual const bool select( Tuple const & t) const = 0;
And your code should also work.
Solution 2
You need to explicitly tell the compiler that your function will not modify any members:
bool const select(Tuple const & tup) const {
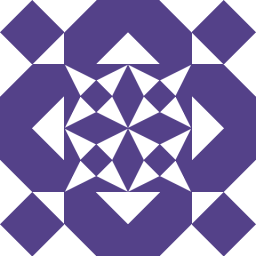
financial_physician
Updated on June 04, 2022Comments
-
financial_physician over 1 year
I'm trying to call a function on a polymorphic item. But I get the following error message at compile time:
'
this
' argument to member function 'select
' has type 'const SelectParam
', but function is not markedconst
the error is shown at the p->selection(*it)
std::set<Tuple>::iterator it; for (it = tuples.begin(); it != tuples.end();) { for (const SelectParam* p: selectionParams) { bool successful = p->select(*it); if( !successful ) { it = tuples.erase(it); } else { it++; } } }
and here's how those classes are defined. (I use to not have all the const and & is there but I put them everywhere I could in hopes that I would make whatever it wanted const but clearly I'm not approaching the problem right since it's not changing anything.
In one of the child classes that is being stored at parent pointer.
bool const select(Tuple const & tup) { bool matched = false; if (tup[idx] == val) { matched = true; } return matched; }
In the other child class that is being used with polymorphism
bool const select(Tuple const & tup) { bool matched = false; if (tup[idx1] == tup[idx2]) { matched = true; } return matched; }
And finally here's the parent class that's super simple.
class SelectParam { public: virtual const bool select( Tuple const & t) = 0; };
Thanks in advance for being willing to help my feeble brain.