5.7.57 SMTP - Client was not authenticated to send anonymous mail during MAIL FROM error
Solution 1
You seem to be passing the From
address as emailAddress
, which is not a proper email address. For Office365 the From
needs to be a real address on the Office365 system.
You can validate that if you hardcode your email address as the From
and your Office 365 password.
Don't leave it there though of course.
Solution 2
@Reshma- In case you have not figured it yet, here are below things that I tried and it solved the same issue.
Make sure that NetworkCredentials you set are correct. For example in my case since it was office SMTP, user id had to be used in the NetworkCredential along with domain name and not actual email id.
You need to set "UseDefaultCredentials" to false first and then set Credentials. If you set "UseDefaultCredentials" after that it resets the NetworkCredential to null.
Hope it helps.
Solution 3
I spent way too much time on this and the solution was super simple. I had to use my "MX" as the host and port 25.
var sClient = new SmtpClient("domain-com.mail.protection.outlook.com");
var message = new MailMessage();
sClient.Port = 25;
sClient.EnableSsl = true;
sClient.Credentials = new NetworkCredential("user", "password");
sClient.UseDefaultCredentials = false;
message.Body = "Test";
message.From = new MailAddress("[email protected]");
message.Subject = "Test";
message.CC.Add(new MailAddress("[email protected]"));
sClient.Send(message);
Solution 4
I use to have the same problem.
Add the domain solved it..
mySmtpClient.Credentials = New System.Net.NetworkCredential("[email protected]", "password", "domain.com")
Solution 5
In my case, 2 Factor Authentication was turned on for the FROM account in Office 365. Once that was turned off, the email sent successfully.
Related videos on Youtube
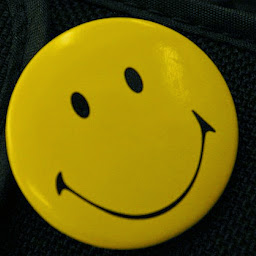
Comments
-
Reshma almost 2 years
I have to send mails using my web application. Given the below code showing
The SMTP server requires a secure connection or the client was not authenticated. The server response was:
5.7.57 SMTP; Client was not authenticated to send anonymous mail during MAIL FROM.
Help me to find a proper solution. Thank you.
Code:
protected void btnsubmit_Click(object sender, EventArgs e) { Ticket_MailTableAdapters.tbl_TicketTableAdapter tc; tc = new Ticket_MailTableAdapters.tbl_TicketTableAdapter(); DataTable dt = new DataTable(); dt = tc.GetEmail(dpl_cate.SelectedValue); foreach (DataRow row in dt.Rows) { string eml = (row["Emp_Email"].ToString()); var fromAddress = "emailAddress"; var toAddress = eml; const string fromPassword = "*****"; string body = "Welcome.."; // smtp settings var smtp = new System.Net.Mail.SmtpClient(); { smtp.Host = "smtp.office365.com"; smtp.Port = 587; smtp.EnableSsl = true; smtp.DeliveryMethod = System.Net.Mail.SmtpDeliveryMethod.Network; smtp.Credentials = new NetworkCredential(fromAddress, fromPassword); smtp.UseDefaultCredentials = false; smtp.Timeout = 600000; } // Passing values to smtp object smtp.Send(fromAddress, toAddress, subject, body); } } }
-
Mahesh Kava about 9 yearswhy not increase the Timeout?
-
fk2 about 9 yearsDid you anonymize this, or are you actually trying to send over office.com with that login?
-
Rishi Jagati over 6 years@reshma, What changes did you make? Facing the same issue.
-
Raphael about 5 yearsI've been ableto send an email with the SMTP config shown, but I moved smtp.UseDefaultCredentials = false; before smtp.Credentials = ... as Kiquenet write below
-
-
nwestfall almost 8 yearsThat's it! I didn't know "UseDefaultCredentials" clears the Credentials. Thanks!
-
EJA over 7 yearsThis is the correct solution for me. I've had an application running, sending daily emails for over a year, suddenly it stopped working. The change to fix it is this one, even though my network credentials aren't actually my email, this fix worked.
-
wajira000 over 7 yearsThis one is worked for me. check this Microsoft article support.office.com/en-us/article/…
-
Simon Price over 6 yearsThis worked for me, but I had to then go to sender.office.com to delist my IP address
-
Muhammad Ali over 6 yearsfor me sClient.EnableSsl = true; was not defined.
-
Kevin .NET over 6 yearsI changed only one time. It told me the password expired when I try to login.
-
devlin carnate over 6 yearswhat language is this? It doesn't appear to be C# SmtpClient()
-
Justinas Jakavonis over 6 yearsIt's properties list which can be parsed in any language.
-
Kiquenet about 6 years
You need to set "UseDefaultCredentials" to false first and then set Credentials. If you set "UseDefaultCredentials" after that it resets the NetworkCredential to null.
-
Kiquenet about 6 yearsHow TLS enable in C# ?
-
Kiquenet about 6 yearsHow you get the MX value ?
-
Kiquenet about 6 yearsWhich is the difference about
domain-com.mail.protection.outlook.com
andsmtp.office365.com
? Can I send to xxx@anotherDomain recipients ? -
Weihui Guo over 5 yearsIn my case, it doesn't matter which one goes first. As long as the "UseDefaultCredentials" is false. It works. I wonder why.
-
Weihui Guo over 5 yearsIt's not adding the domain name, but using the email address as the userName solved my problem.
NetworkCredential(email, password);
-
Lez over 5 yearsUsing the MX record as host enables you to send email only to internal email addresses (ie only to the same domain as the sending address)
-
Abdus Salam Azad about 5 yearsThis is not like C# SmtpClient()
-
Martijn over 3 years@Kiquenet You may need to ask your system administrator. More info here: docs.microsoft.com/en-us/exchange/mail-flow-best-practices/…
-
Kiquenet over 3 yearsHow is 2 Factor Authentication ?
-
West about 3 yearsFinally a working answer for me. Thanks! I was using a simple string for the To field instead of type MailAddress. The error message is definitely misleading
-
Chirag about 2 yearsStep 1 worked for me. I had Authenticated SMTP unchecked. When checked and saved, emails started working through my CSharp program.