A circular reference has been detected in Symfony
10,389
Solution 1
You need to call $normalizer->setCircularReferenceHandler()
please read official documentation below:
Solution 2
$jsonContent = $serializer->serialize($yourObject, 'json', [
'circular_reference_handler' => function ($object) {
return $object->getId();
}
]);
Above Works for me. Hope this will be helpfull (Symfony >=4.2)
Related videos on Youtube
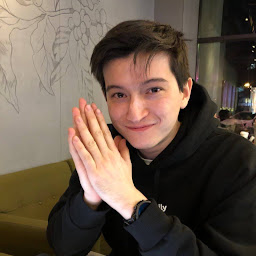
Author by
Ruslan Poltaev
Updated on June 23, 2022Comments
-
Ruslan Poltaev almost 2 years
I run into problem tied to circular reference in Symfony, that I suspect to be caused by the serializer but I haven't found any answers yet. Here are the entities that I've created, the route and the controller. Any suggestions in this regard will be much appreciated.
User.php
class User { /** * @var int * * @ORM\Column(name="id", type="integer") * @ORM\Id * @ORM\GeneratedValue(strategy="AUTO") */ private $id; /** * @ORM\OneToMany(targetEntity="Dieta", mappedBy="user") */ private $dietas; public function __construct() { $this->dietas = new ArrayCollection(); } //... //... }
Dieta.php
class Dieta { /** * @var int * * @ORM\Column(name="id", type="integer") * @ORM\Id * @ORM\GeneratedValue(strategy="AUTO") */ private $id; /** * @ORM\ManyToOne(targetEntity="User", inversedBy="dietas") * @ORM\JoinColumn(name="users_id", referencedColumnName="id") */ private $user; public function __construct() { $this->user = new ArrayCollection(); } //... //... }
Route
/** * @Route("dietas/list/user/{id}", name="userDietas") */
Method of DietaController.php
public function userListAction($id) { $encoders = array(new XmlEncoder(), new JsonEncoder()); $normalizers = array(new ObjectNormalizer()); $serializer = new Serializer($normalizers, $encoders); $user = $this->getDoctrine() ->getRepository('AppBundle:User')->find($id); $dietaDatas = $user->getDietas(); if(!$dietaDatas) { throw $this->createNotFoundException( 'There is no data...' ); } $jsonContent = $serializer->serialize($dietaDatas, 'json'); return new Response($jsonContent); }