a server side mustache.js example using node.js
25,693
Solution 1
I got your example working by installing mustache via npm, using the correct require syntax and (as Derek said) using mustache as an object not a function
npm install mustache
then
var sys = require('sys');
var mustache = require('mustache');
var view = {
title: "Joe",
calc: function() {
return 2 + 4;
}
};
var template = "{{title}} spends {{calc}}";
var html = mustache.to_html(template, view);
sys.puts(html);
Solution 2
Your example is almost correct. Mustache is an object, not a function, so it doesn't need the (). Rewritten as
var html = Mustache.to_html(template, view);
will make it happier.
Solution 3
Thanks to Boldr http://boldr.net/create-a-web-app-with-node Had to add the following code to mustache.js
for (var name in Mustache)
if (Object.prototype.hasOwnProperty.call(Mustache, name))
exports[name] = Mustache[name];
Not exactly sure what it is doing but it works. Will try to understand it now.
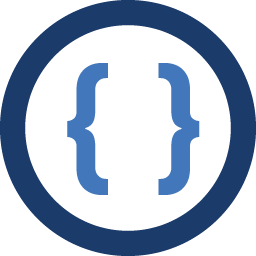
Author by
Admin
Updated on January 19, 2020Comments
-
Admin over 4 years
I'm looking for an example using
Mustachejs
withNodejs
here is my example but it is not working.
Mustache
is undefined. I'm using Mustachejs from the master branch.var sys = require('sys'); var m = require("./mustache"); var view = { title: "Joe", calc: function() { return 2 + 4; } }; var template = "{{title}} spends {{calc}}"; var html = Mustache().to_html(template, view); sys.puts(html);