Accessing ResourceDictionary from WPF UserControl
Solution 1
To access one of your UserControl's XAML resources in your codebehind, all you need to do is access the Resources property of the UserControl. Something like this:
BitmapImage myImage = (BitmapImage)this.Resources["imageDefault"];
Though, the preferred method is to use FindResource(), which will search the entire logical tree for a match to the key, rather than just the object it is called on.
BitmapImage myImage = (BitmapImage)this.FindResource("imageDefault");
Solution 2
Try to remove the forward slash infront of your location. The only time you should use /Resources is if you have to go up a library first. like ../Resources
<UserControl.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="Resources/BiometricDictionary.xaml" />
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
Hope this helps you.
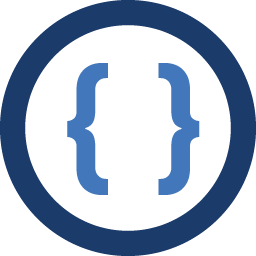
Admin
Updated on April 16, 2020Comments
-
Admin about 4 years
I'm trying to access a resource dictionary in a UserControl code-behind via C# and I'm having little success.
Merged Dictionary:
<UserControl.Resources> <ResourceDictionary> <ResourceDictionary.MergedDictionaries> <ResourceDictionary Source="/Resources/BiometricDictionary.xaml" /> </ResourceDictionary.MergedDictionaries> </ResourceDictionary> </UserControl.Resources>
Embedded Dictionary:
<UserControl.Resources> <BitmapImage x:Key="imageDefault">/Resources/Images/default_thumb.png</BitmapImage> <BitmapImage x:Key="imageDisconnected">/Resources/Images/disconnect_thumb.png</BitmapImage> <BitmapImage x:Key="imageFailed">/Resources/Images/failed_thumb.png</BitmapImage> <BitmapImage x:Key="imageSuccess">/Resources/Images/success_thumb.png</BitmapImage> </UserControl.Resources>
Code behind:
var resourceDictionary = new ResourceDictionary(); resourceDictionary.Source = new Uri("/Resources/BiometricDictionary.xaml", UriKind.Relative);
I've tried all of the examples and helpful tips but coming up short. Right now, success would be the ability to load the dictionary. Any suggestions?