Add Access-Control-Allow-Origin: * easily to JSON
Solution 1
Option 1: Use PHP
You can't add it to the .json file. You would need to create a php file which returns the JSON data. A crude example might be:
/json/index.php?f=foo
header("Access-Control-Allow-Origin: *");
header("content-type: application/json");
echo file_get_contents($_REQUEST['f'].".json");
This will allow you to set the Access-Control-Allow-Origin header and return the desired json file content to your remote call:
$.getJSON("http://foo.com/json/index.php?f=foo", function(json) {
console.log(json);
});
Option 2: Use Server Configuration
Another option would be to configure the header to apply to json files in your server config. Using Apache2 you could add the following to your server config or create a .htaccess file in the /json directory to include:
<Files "*.json">
Header set Access-Control-Allow-Origin "*"
</Files>
This would include the header for all the json files automatically.
Solution 2
Don't access the JSON file directly, use a PHP page in the middle. So you call www.foo.com/json/, which will run the default PHP file in that folder (say index.php). Now this PHP page will set the CORS header like you mentioned:
header("Access-Control-Allow-Origin: *");
and then read the JSON file and add it to the response along with the correct MIME type:
header("content-type: application/json");
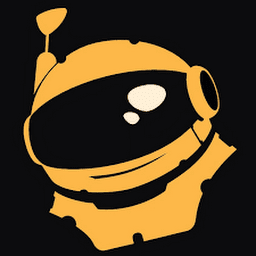
johnpyp
Updated on June 09, 2022Comments
-
johnpyp almost 2 years
On my web server I have a folder (say the link is: www.foo.com/json/).
Inside this link, I have a json file, like foo.json. I want to access this file from another website. I've tried to research "CORS" but it seems pretty complicated, and I don't use any backend in on my website foo.com. I've heard you can simply use php and put this in:
<?php header("Access-Control-Allow-Origin: *");
How do I add this to that folder that has the .json so that when I do:
$.getJSON("http://foo.com/json/foo.json", function(json) { console.log(json )});
It won't throw the error. I want this to be simple to let any website do it, I just don't know how to connect it all.
-
johnpyp almost 7 yearsAwesome, it works great! Thanks for the cleanest explanation I've found :) Can't upvote because I don't have the reputation.
-
johnpyp almost 7 yearsQuestion: Could someone theoretically POST to the json since I allowed Access-Control-Allow-Origin? Or is this read-only?
-
M31 almost 7 yearsIf you mean to ask if the JSON files can be modified then I think this would only be possible using the php method if you were to add code to handle input data and write to the file in index.php. The Access-control-allow-origin header is only used to allow the use of resources remotely what can be done with those resources is no different than accessing them locally and is based on the server configuration and services facilitating those actions.