Add an implementation, such as Hibernate Validator, to the classpath error using springboot
Solution 1
You are missing some libs. Add the following Spring Boot dependency to your pom.xml
to solve it. Spring Boot will find out the correct implementations to use in your project.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
Also, add Hibernate Dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- Hibernate -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-ehcache</artifactId>
<exclusions>
<exclusion>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-java8</artifactId>
</dependency>
Solution 2
There should be fixed in the latest spring boot versions per this link: https://github.com/spring-projects/spring-boot/pull/12669 (Allow validation api in the classpath without an implementation). In case of older boot versions, another option to fix this exception is to exclude the vaidation API. Example below when using spring-boot-starter web:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>javax.validation</groupId>
<artifactId>validation-api</artifactId>
</exclusion>
</exclusions>
</dependency>
Solution 3
I only needed to add:
compile 'org.springframework.boot:spring-boot-starter-validation'
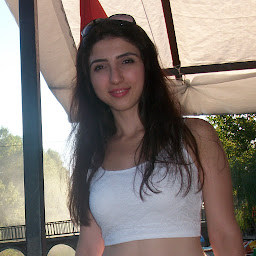
nurdan karaman
Updated on July 22, 2022Comments
-
nurdan karaman almost 2 years
I m new to Spring Boot and built my first simple project but have a problem when running the application.
Could you please tell me why it s giving an error , below?
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>io.javabrains.springbootquickstart</groupId> <artifactId>course-api</artifactId> <version>0.0.1-SNAPSHOT</version> <name>java Brains Course API</name> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.1.RELEASE</version> </parent> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-validator</artifactId> </dependency> </dependencies> <properties> <java.version>1.8</java.version> </properties> </project>
CourseApiApp.java
package io.javabrains.springbootstarter; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class CourseApiApp { public static void main(String[] args) { SpringApplication.run(CourseApiApp.class, args); } }
This screenshot belongs my example.
Console output below: Application failed to start. "Add an implementation, such as Hibernate Validator, to the classpath"
. ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v1.5.1.RELEASE) 2018-01-09 11:31:47.216 INFO 12224 --- [ main] i.j.springbootstarter.CourseApiApp : Starting CourseApiApp on kafein-kafein with PID 12224 (C:\Users\kafein\Documents\workspace-sts-3.9.2.RELEASE\course-api\target\classes started by kafein in C:\Users\kafein\Documents\workspace-sts-3.9.2.RELEASE\course-api) 2018-01-09 11:31:47.223 INFO 12224 --- [ main] i.j.springbootstarter.CourseApiApp : No active profile set, falling back to default profiles: default 2018-01-09 11:31:47.314 INFO 12224 --- [ main] s.c.a.AnnotationConfigApplicationContext : Refreshing org.springframework.context.annotation.AnnotationConfigApplicationContext@2a8448fa: startup date [Tue Jan 09 11:31:47 EET 2018]; root of context hierarchy WARNING: An illegal reflective access operation has occurred WARNING: Illegal reflective access by org.springframework.cglib.core.ReflectUtils$1 (file:/C:/Users/kafein/.m2/repository/org/springframework/spring-core/4.3.6.RELEASE/spring-core-4.3.6.RELEASE.jar) to method java.lang.ClassLoader.defineClass(java.lang.String,byte[],int,int,java.security.ProtectionDomain) WARNING: Please consider reporting this to the maintainers of org.springframework.cglib.core.ReflectUtils$1 WARNING: Use --illegal-access=warn to enable warnings of further illegal reflective access operations WARNING: All illegal access operations will be denied in a future release 2018-01-09 11:31:48.148 WARN 12224 --- [ main] s.c.a.AnnotationConfigApplicationContext : Exception encountered during context initialization - cancelling refresh attempt: org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'standardJacksonObjectMapperBuilderCustomizer' defined in class path resource [org/springframework/boot/autoconfigure/jackson/JacksonAutoConfiguration$Jackson2ObjectMapperBuilderCustomizerConfiguration.class]: Unsatisfied dependency expressed through method 'standardJacksonObjectMapperBuilderCustomizer' parameter 1; nested exception is org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'spring.jackson-org.springframework.boot.autoconfigure.jackson.JacksonProperties': Initialization of bean failed; nested exception is javax.validation.ValidationException: Unable to create a Configuration, because no Bean Validation provider could be found. Add a provider like Hibernate Validator (RI) to your classpath. 2018-01-09 11:31:48.156 INFO 12224 --- [ main] utoConfigurationReportLoggingInitializer : Error starting ApplicationContext. To display the auto-configuration report re-run your application with 'debug' enabled. 2018-01-09 11:31:48.162 ERROR 12224 --- [ main] o.s.b.d.LoggingFailureAnalysisReporter : *************************** APPLICATION FAILED TO START *************************** Description: The Bean Validation API is on the classpath but no implementation could be found Action: Add an implementation, such as Hibernate Validator, to the classpath