Add custom order status and send email on status change in Woocommerce
New version code: Add a new order status that Send an email notification in WooCommerce 4+
To make it work for a new custom order status (here "Awaiting delivery") you should need:
- to register the custom new order status first,
- to display it in bulk edit dropdown on admin orders list (optional),
- to display it in order edit pages status dropdown
- to send an customized email notification when an order get this custom status.
The code:
// register a custom post status 'awaiting-delivery' for Orders
add_action( 'init', 'register_custom_post_status', 20 );
function register_custom_post_status() {
register_post_status( 'wc-awaiting-delivery', array(
'label' => _x( 'Awaiting delivery', 'Order status', 'woocommerce' ),
'public' => true,
'exclude_from_search' => false,
'show_in_admin_all_list' => true,
'show_in_admin_status_list' => true,
'label_count' => _n_noop( 'Awaiting delivery <span class="count">(%s)</span>', 'Awaiting delivery <span class="count">(%s)</span>', 'woocommerce' )
) );
}
// Adding custom status 'awaiting-delivery' to order edit pages dropdown
add_filter( 'wc_order_statuses', 'custom_wc_order_statuses', 20, 1 );
function custom_wc_order_statuses( $order_statuses ) {
$order_statuses['wc-awaiting-delivery'] = _x( 'Awaiting delivery', 'Order status', 'woocommerce' );
return $order_statuses;
}
// Adding custom status 'awaiting-delivery' to admin order list bulk dropdown
add_filter( 'bulk_actions-edit-shop_order', 'custom_dropdown_bulk_actions_shop_order', 20, 1 );
function custom_dropdown_bulk_actions_shop_order( $actions ) {
$actions['mark_awaiting-delivery'] = __( 'Mark Awaiting delivery', 'woocommerce' );
return $actions;
}
// Adding action for 'awaiting-delivery'
add_filter( 'woocommerce_email_actions', 'custom_email_actions', 20, 1 );
function custom_email_actions( $action ) {
$actions[] = 'woocommerce_order_status_wc-awaiting-delivery';
return $actions;
}
add_action( 'woocommerce_order_status_wc-awaiting-delivery', array( WC(), 'send_transactional_email' ), 10, 1 );
// Sending an email notification when order get 'awaiting-delivery' status
add_action('woocommerce_order_status_awaiting-delivery', 'backorder_status_custom_notification', 20, 2);
function backorder_status_custom_notification( $order_id, $order ) {
// HERE below your settings
$heading = __('Your Awaiting delivery order','woocommerce');
$subject = '[{site_title}] Awaiting delivery order ({order_number}) - {order_date}';
// Getting all WC_emails objects
$mailer = WC()->mailer()->get_emails();
// Customizing Heading and subject In the WC_email processing Order object
$mailer['WC_Email_Customer_Processing_Order']->heading = $heading;
$mailer['WC_Email_Customer_Processing_Order']->subject = $subject;
// Sending the customized email
$mailer['WC_Email_Customer_Processing_Order']->trigger( $order_id );
}
Code goes in function.php file of your active child theme (or active theme).
Tested and works (should work on any Woocommerce versions above 2.5)
In others function if you use the WC_Order method
update_status()
to change an order to 'awaiting-delivery' status like:$order->update_status();
the related email notification will be sent too.
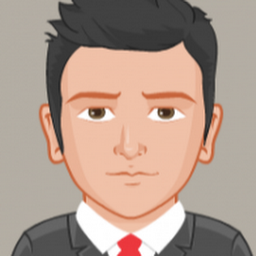
shruti kharge
Updated on June 25, 2022Comments
-
shruti kharge almost 2 years
I want to add custom order status in my woocommerce site.And whenever status changes to that custom status I want to send mail.
I have tried Send an email notification when custom order status changes in WooCommerce
as well as https://github.com/sarun007/custom-email-plugin/tree/master
But didn't worked
I am using woocommerce 3.2.6 version -
shruti kharge over 6 yearsThanks Loic,I will try this one
-
shruti kharge over 6 yearsi have tried,but its not working.it adds status in drop down but it does not set that in email settings
-
LoicTheAztec over 6 years@ShrutiKharge The answer here is just answering your initial question. To add a new email notification in backend for a custom order status, is just another question that has nothing to do with what you asked here.
-
TobiasM over 4 yearsthis works great and is working perfectly fine. However, I was wondering if it's possible to change the body text of the email as well? Just like the snippet is using $heading and $subject is it then possible to use $message_body or something similar?
-
LoicTheAztec over 4 years@TobiasM I don't think so… In this case you should need something different and more complicated with a custom email notification.