Add element to null (empty) List<T> Property
Solution 1
Add element to null (empty) List Property
null
and an empty list are two different things: Adding an element to an empty list works fine, but if your property is null
(as all reference-type properties are initially null
), you need to initialize it with an empty list first.
You could use an auto-property initializer for that (see Kędrzu's answer), or you could manually initialize the list in the constructor:
class Maps
{
public Maps()
{
AllAntsAtMap = new List<Ant>();
}
...
}
(Since the property is declared in the superclass Maps, I'd do the initialization there rather than in the subclass Quadrangle.)
Solution 2
It is much simpler in C# 6:
protected List<Ant> AllAntsAtMap { get; set; } = new List<Ant>();
Solution 3
You should initialize AllAntsAtMap
before usage. You can use the constructor for that:
public Quadrangle()
{
AllAntsAtMap = new List<Ant>();
}
Solution 4
You need to instantiate the list before adding:
this.AllAntsAtMap = new List<AllAntsAtMap>();
Solution 5
Initialise the list in the constructor.
class Maps
{
protected virtual List<Ant> AllAntsAtMap { get; set; }
public Maps()
{
AllAntsAtMap = new List<Ant>();
}
}
You don't need to override the list property in Quadrangle
unless you specifically need a separate list from the base class. If you do have a separate list in the child class then you need to initialise that list in the child class constructor.
If the base class shouldn't have an implementation of the list property, then you should make it abstract
instead of virtual
, and initialise it in the child class where the property is implemented.
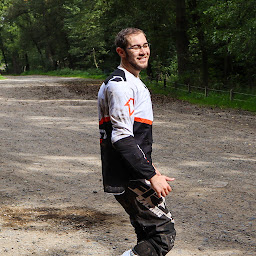
Sinmson
Updated on July 05, 2022Comments
-
Sinmson almost 2 years
I got a problem.
The problem is that I try to ad an object to a list of this objects. This list is a property, no error, but when I run it fails at this point, becouse: "NullReferenceException". Sounds logical, becouse the Property of the list is "null", but I cant declare a property, can I?
Her is some Code snipped:
class Maps { protected virtual List<Ant> AllAntsAtMap { get; set; }
[...]
class Quadrangle : Maps { protected override List<Ant> AllAntsAtMap { get; set; } public override void AddAntToMap(Ant ant) { AllAntsAtMap.Add(ant); //Error here } public override void AddAntsToMap(List<Ant> ants) { foreach (Ant ant in ants) { AddAntToMap(ant); } }
[...]
-
siride over 8 yearsCool, but they probably aren't using C# 6.
-
Kędrzu over 8 yearsHow this assumption is this related to question?
-
Joe Johnston over 3 years5 years later: Both methods work in .NET Core 3.1 (This and init in the ctor)