Add Image to JOptionPane
48,611
Solution 1
JOptionPane
is a very flexible API.
Your first port of call should be the Java API Docs and the Java Trails, specific How to use Dialogs
public class TestOptionPane04 {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
}
ImageIcon icon = new ImageIcon(TestOptionPane04.class.getResource("/earth.png"));
JOptionPane.showMessageDialog(
null,
"Hello world",
"Hello", JOptionPane.INFORMATION_MESSAGE,
icon);
JOptionPane.showMessageDialog(
null,
new JLabel("Hello world", icon, JLabel.LEFT),
"Hello", JOptionPane.INFORMATION_MESSAGE);
}
});
}
}
Solution 2
From the javadoc on JOptionPane:
public static void showMessageDialog(Component parentComponent,
Object message,
String title,
int messageType,
Icon icon)
throws HeadlessException
Just make an Icon
of your image and add it as a 5th parameter.
JOptionPane.showMessageDialog(null, textArea, "Border States",
JOptionPane.PLAIN_MESSAGE, image2);
Don't forget to define image2 before you use it (move the line up)
Solution 3
What is a MessageDialogBox? If you mean add an image to a JOptionPane, there are method overloads that accept an Icon, and so this is one way to solve this. Another is to create a JPanel or JLabel with your image and other components and then display this in the JOptionPane.
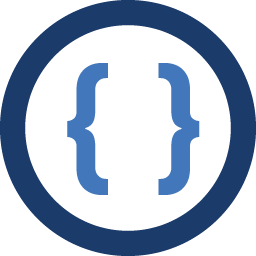
Author by
Admin
Updated on December 20, 2020Comments
-
Admin over 3 years
I'm wondering How To Add Image To MessageDialog Box. I tried the code below and the image was nowhere to be found
else if(button == B){ String text = "blahblahblahblahblah"; JTextArea textArea = new JTextArea(text); textArea.setColumns(30); textArea.setLineWrap( true ); textArea.setWrapStyleWord( true ); textArea.setSize(textArea.getPreferredSize().width, 1); Font font = new Font("Verdana", Font.BOLD, 12); textArea.setFont(font); textArea.setForeground(Color.BLUE); JOptionPane.showMessageDialog( null, textArea, "Border States", JOptionPane.PLAIN_MESSAGE); image2 = new ImageIcon(getClass().getResource("borderstates.jpg")); label2 = new JLabel(image2); add(label2);