Add string to string in C with safe functions
16,590
Solution 1
The size
parameter of the _s functions is the size of the destination buffer, not the source. The error is because there is no null terminator in nieuw
in the first for characters. Try this:
size = strlen(locatie);
size++;
int nieuwSize = size + 4;
nieuw = (char*)malloc(nieuwSize );
strcpy_s(nieuw, nieuwSize, locatie);
nieuw[size] = '\0';
strcat_s(nieuw, nieuwSize, ".cpt"); // <-- crash
puts(nieuw);
Solution 2
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(void)
{
char old[] = "hello";
size_t size = strlen(old) + 5;;
char *new_name = (char*)malloc(size);
new_name[size-1] = '\0';
strcpy_s(new_name, size, old);
strcat_s(new_name, size, ".cpp");
printf("%s\n", new_name);
return 0;
}
Solution 3
Maybe some standard solution?
const char * const SUFFIX = ".cpt";
size = strlen(locatie) + strlen(SUFFIX) + 1; // +1 for NULL
nieuw = (char*)malloc(size);
snprintf(nieuw, size, "%s%s", locatie, SUFFIX);
Related videos on Youtube
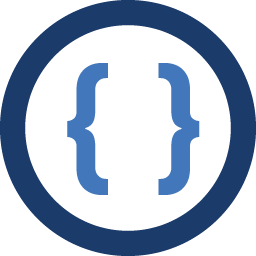
Author by
Admin
Updated on July 30, 2022Comments
-
Admin over 1 year
I want to copy the a file name to a string and append ".cpt" to it. But I am unable to do this with safe functions (strcat_s). Error: "String is not null terminated!". And I did set '\0', how to fix this using safe functions?
size = strlen(locatie); size++; nieuw = (char*)malloc(size+4); strcpy_s(nieuw, size, locatie); nieuw[size] = '\0'; strcat_s(nieuw, 4, ".cpt"); // <-- crash puts(nieuw);
-
Admin over 11 yearsasprintf is not available for windows.
-
another.anon.coward over 11 years+1, Though I have no experience with MS function just curious after reading documentation of strcpy_s, isn't
nieuw[size] = '\0';
redundant? -
shf301 over 11 yearsYes it is redundant.
strcpy_s
will always null terminate the string. -
kakyo over 4 yearsmuch nicer. I can't believe how much harder it is to get
strcat_s
working right.