Adding an onclick event to a table row
Solution 1
Something like this.
function addRowHandlers() {
var table = document.getElementById("tableId");
var rows = table.getElementsByTagName("tr");
for (i = 0; i < rows.length; i++) {
var currentRow = table.rows[i];
var createClickHandler = function(row) {
return function() {
var cell = row.getElementsByTagName("td")[0];
var id = cell.innerHTML;
alert("id:" + id);
};
};
currentRow.onclick = createClickHandler(currentRow);
}
}
EDIT
Working demo.
Solution 2
I think for IE you will need to use the srcElement property of the Event object. if jQuery is an option for you, you may want to consider using it - as it abstracts most browser differences for you. Example jQuery:
$("#tableId tr").click(function() {
alert($(this).children("td").html());
});
Solution 3
Simple way is generating code as bellow:
<!DOCTYPE html>
<html>
<head>
<style>
table, td {
border:1px solid black;
}
</style>
</head>
<body>
<p>Click on each tr element to alert its index position in the table:</p>
<table>
<tr onclick="myFunction(this)">
<td>Click to show rowIndex</td>
</tr>
<tr onclick="myFunction(this)">
<td>Click to show rowIndex</td>
</tr>
<tr onclick="myFunction(this)">
<td>Click to show rowIndex</td>
</tr>
</table>
<script>
function myFunction(x) {
alert("Row index is: " + x.rowIndex);
}
</script>
</body>
</html>
Solution 4
Here is a compact and a bit cleaner version of the same pure Javascript (not a jQuery) solution as discussed above by @redsquare and @SolutionYogi (re: adding onclick
event handlers to all HTML table rows) that works in all major Web Browsers, including the latest IE11:
function addRowHandlers() {
var rows = document.getElementById("tableId").rows;
for (i = 0; i < rows.length; i++) {
rows[i].onclick = function(){ return function(){
var id = this.cells[0].innerHTML;
alert("id:" + id);
};}(rows[i]);
}
}
window.onload = addRowHandlers();
Working DEMO
Note: in order to make it work in IE8 as well, instead of this
pointer use the explicit identifier like function(myrow)
as suggested by @redsquare.
Best regards,
Solution 5
Head stuck in jq for too long. This will work.
function addRowHandlers() {
var table = document.getElementById("tableId");
var rows = table.getElementsByTagName("tr");
for (i = 1; i < rows.length; i++) {
var row = table.rows[i];
row.onclick = function(myrow){
return function() {
var cell = myrow.getElementsByTagName("td")[0];
var id = cell.innerHTML;
alert("id:" + id);
};
}(row);
}
}
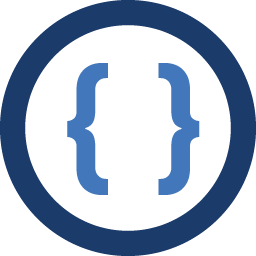
Admin
Updated on January 24, 2022Comments
-
Admin over 2 years
I'm trying to add an onclick event to a table row through Javascript.
function addRowHandlers() { var table = document.getElementById("tableId"); var rows = table.getElementsByTagName("tr"); for (i = 1; i < rows.length; i++) { row = table.rows[i]; row.onclick = function(){ var cell = this.getElementsByTagName("td")[0]; var id = cell.innerHTML; alert("id:" + id); }; } }
This works as expected in Firefox, but in Internet Explorer (IE8) I can't access the table cells. I believe that is somehow related to the fact that "this" in the onclick function is identified as "Window" instead of "Table" (or something like that).
If I could access the the current row I could perform a
getElementById
in the onclick function by I can't find a way to do that. Any suggestions?