Adding compiled libraries and include files to a CMake Project?
Solution 1
Recent versions already have a module for finding FreeType. Here's the kind of thing I've done in the past:
INCLUDE(FindFreetype)
IF(NOT FREETYPE_FOUND)
FIND_LIBRARY(FREETYPE_LIBRARIES NAMES libfreetype freetype.dll PATHS "./libs/MacOS" "./libs/Windows" DOC "Freetype library")
FIND_PATH(FREETYPE_INCLUDE_DIRS ftbuild.h "./includes" DOC "Freetype includes")
ENDIF(NOT FREETYPE_FOUND)
INCLUDE_DIRECTORIES(${FREETYPE_INCLUDE_DIRS})
TARGET_LINK_LIBRARIES(MyProject ${FREETYPE_LIBRARIES})
You'll need to change the paths to be relative to your CMakeLists.txt.
This snippet first invokes the FindFreetype module to check in the standard system locations. If it fails to find the library there, then this falls back to checking directories relative to the your CMakeLists.txt script. If that still fails, you can still set or override the locations via the usual CMake UI. In any event, it tries to add something to the list of includes and libraries to link.
Solution 2
Just use target_link_libraries with the full path to the prebuilt lib.
So, something like:
# In the file Source/MyProject/CMakeLists.txt
add_executable(my_exe main.cpp foo.cpp foo.h)
if(WIN32)
target_link_libraries(my_exe ${CMAKE_CURRENT_SOURCE_DIR}/../libs/Windows/freetype.lib)
endif()
if(APPLE)
target_link_libraries(my_exe ${CMAKE_CURRENT_SOURCE_DIR}/../libs/MacOS/libfreetype.a)
endif()
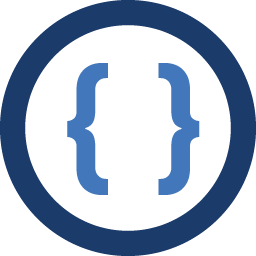
Admin
Updated on April 09, 2020Comments
-
Admin about 4 years
What is the best method to include a prebuilt library to a cmake project? I want to include FreeType into the project I am working on and the file structure is like this:
- Build
- MacOS
- Make/
- XCode/
- Windows
- VisualStudio/
- MacOS
- Source
- libs
- MacOS
- libfreetype
- Windows
- freetype.dll
- MacOS
- includes
- freetype/ (Various header files that are included automatically by ftbuild.h)
- ftbuild.h (this is what is included in code from my understanding.)
- MyProject
- main.cpp
- foo.cpp
- foo.h
- libs
The library is already compiled. MyProject is the name of the current project.
Thanks! Mike
- Build