Adding Event Listeners in constructor
10,793
Solution 1
Your this
value changes in the constructor. You can keep a reference in the selector, and use the reference.
function Foo(elementId, buttonId) {
/*...*/
var self = this;
this.button.addEventListener('click', function(e) {self.bar();}, false);
}
Or a more modern solution that doesn't require a variable would be to use Function.prototype.bind
.
function Foo(elementId, buttonId) {
/*...*/
this.button.addEventListener('click', this.bar.bind(this), false);
}
The .bind
method returns a new bar
function with the this
value bound to whatever you passed it. In this case, it is bound to the original this
from the constructor.
Solution 2
this
in the event handler is the element that the event was added to.
To access the outer this
, you need to store it in a separate variable.
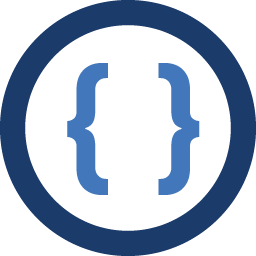
Author by
Admin
Updated on July 20, 2022Comments
-
Admin almost 2 years
function Foo(elementId, buttonId) { this.element = document.getElementById(elementId); this.button = document.getElementById(buttonId); this.bar = function() {dosomething}; this.button.addEventListener('click', function(e) {this.bar();}, false); } var myFoo = new Foo('someElement', 'someButton');
I'd like to add event listeners inside my constructor, but it doesn't seem to work. Is this something that's possible with the correct syntax? I always get hung up on the line:
this.button.addEventListener('click', function(e) {this.bar();}, false);
-
zachelrath over 11 yearsImportant to note that many browsers still commonly-used (e.g. IE 8, Safari <= 5.1, others) do not support Function.prototype.bind: kangax.github.com/es5-compat-table
-
Admin over 11 yearsvar self = this; That did it; I didn't even think that 'this' might be referencing the button element. Thanks for your help and the quick response. I'd upvote if I could, but I don't have the rep yet :)
-
I Hate Lazy over 11 years@Llepwryd: Glad I could help.