Addition and subtraction of complex numbers using class in C++
The line
cout << "The sum is " << result.add << endl;
is incorrect, as add
is a method so result.add
will be a pointer to that method, and cout
does not know how to handle it - which makes the compiler spit it out.
Change the line to
cout << "The sum is ";
result.print();
cout << endl;
You need to do the same for the line
cout << "The difference is " << result.subtract << endl;
As to coding style, the two methods are overwrting an existing complex number. Perhaps having a the function like this would be better
Complex &Complex::add (const Complex &op) {
r += op.r;
i += op.i;
return *this;
}
This will enable you to chain additions together and also just add a complex number to the existing complex number.
In addition you could make the class variables r
and i
private. This will require an alternative constructor:
Complex:Complex(double real, double imaginary) : r(real), i(imaginary) {};
Finally you may wish to consider operator overloading - I am sure you can google that to find a reasonable tutorial.
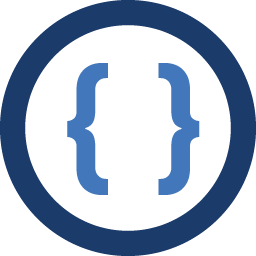
Admin
Updated on July 07, 2022Comments
-
Admin almost 2 years
I have here a code that is supposed to ask the user two sets of real and imaginary numbers.
#include <iostream> using namespace std; class Complex { public: double r; double i; public: Complex(); void add(Complex, Complex); void subtract(Complex, Complex); void print(); }; Complex::Complex() { r = i = 0; } void Complex::add (Complex op1, Complex op2) { r = op1.r+op2.r; i = op1.i+op2.i; } void Complex::subtract (Complex op1, Complex op2) { r = op1.r-op2.r; i = op1.i-op2.i; } void Complex::print () { cout << r << i; } int main () { Complex operand1, operand2, result; cout << "Input real part for operand one: " << endl; cin >> operand1.r; cout << "Input imaginary part for operand one: " << endl; cin >> operand1.i; cout << "Input real part for operand two: " << endl; cin >> operand2.r; cout << "Input imaginary part for operand two: " << endl; cin >> operand2.i; result.add(operand1, operand2); cout << "The sum is " << result.add << endl; result.subtract(operand1, operand2); cout << "The difference is " << result.subtract << endl; }
However, when I compiled the program, lots of errors are displayed (std::basic_ostream) which I don't even get.
Another issue I'm having is in the function void::Complex print. There should be a condition inside cout itself. No if-else. But I have no idea what to do.
The program must run like this:
Input real part for operand one: 5
Input imaginary part for operand one: 2 (the i for imaginary shouldn't be written)
Input real part for operand two: 8
Input imaginary part for operand two: 1 (again, i shouldn't be entered)
/then it will print the input(ed) numbers/
(5, 2i) //this time with an i
(8, 1i)
/then the answers/
The sum is 13+3i.
The difference is -3, 1i. //or -3, iPlease help me! I'm new in C++ and here in stackoverflow and your help would be very appreciated. Thank you very much!
-
penartur about 12 yearsIs this your school homework?
-
Alexander about 12 yearsRead some more about operator overloading and you should be able to write your add and subtract functions properly.
-
Admin about 12 yearsYes, penartur. I think I did what I can but my knowledge is still lacking. I need guidance.
-
slezica about 12 yearsWhich compiler are you using? g++ can be quite cryptic. Maybe try clang++? If not, google individual errors. Put some spirit into it :D
-
Admin about 12 yearsHello, upside down! I use CodeBlocks. Thank you!
-
Douglas Daseeco about 7 yearsWhy reinvent the wheel? stackoverflow.com/questions/42833241/…
-