Adjacent JSX elements must be wrapped in an enclosing tag
Solution 1
When you wrap it in a view make sure you set the flex to 1. My guess is that the view you are giving it has no size and therefore the child elements are inheriting their size from the parent (which is 0)
Solution 2
As per React 16, if you wan't to avoid the <View>
wrapping,
you can return multiple components from render as an array.
return ([
<Navigator key="navigator" />,
<NavBar key="navbar" />
]);
Or in a stateless ES6 component:
import React from 'react';
const Component = () => [
<Navigator key="navigator" />,
<NavBar key="navbar" />
];
export default Component;
Don't forget the key property as React needs (as for iteration on Array) to be able to discriminate your components.
Edit (Nov. 2018)
Your can also use React.Fragment
shorthand:
render() {
return (
<>
<ChildA />
<ChildB />
<ChildC />
</>
);
}
Solution 3
Wrap both inside a View and give that outer View wrapper a style of flex 1. Example:
<View style={{flex: 1}}>
<Navigator
{. . .}
/>
<NavBar />
</View>
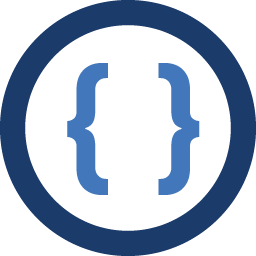
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I want to have a navigation bar at the bottom and a toolbar at the top of every page in my React-Native app. However, if I create these two elements in the index.ios.js file, I get the error: "Adjacent JSX elements must be wrapped in an enclosing tag". If I put tags surrounding the Navigator and NavBar I just see a blank screen in my app. What should I do? Here is what my render function looks like in index.ios.js
render() { return ( <Navigator initialRoute={{name: 'Login'}} renderScene={this.renderScene} navigationBar={ <Navigator.NavigationBar style={ styles.nav } routeMapper={ NavigationBarRouteMapper } /> } /> <NavBar /> ); }