AlarmManager setRepeating()
Solution 1
use this code
AlarmManager alarmMgr;
PendingIntent pendingIntent;
public void startAlarmManager()
{
Intent dialogIntent = new Intent(getBaseContext(), AlarmBroadcastReceiver.class);
alarmMgr = (AlarmManager) this.getSystemService(Context.ALARM_SERVICE);
pendingIntent = PendingIntent.getBroadcast(this, 0, dialogIntent,PendingIntent.FLAG_CANCEL_CURRENT);
alarmMgr.setInexactRepeating(AlarmManager.RTC_WAKEUP,System.currentTimeMillis(), 10000, pendingIntent);
}
}
wheather you want to stop alarm
public void stopAlarmManager()
{
if(alarmMgr != null)
alarmMgr.cancel(pendingIntent);
}
Be Remembered dont forget to register Receiver in manifest file
<receiver android:name=".AlarmBroadcastReceiver" >
</receiver>
Solution 2
Use setInexactRepeating() instead of setRepeating(). When you use setInexactRepeating(), Android synchronizes repeating alarms from multiple apps and fires them at the same time.
This reduces the total number of times the system must wake the device, thus reducing drain on the battery. As of Android 4.4 (API Level 19), all repeating alarms are inexact.
Note that while setInexactRepeating() is an improvement over setRepeating(), it can still overwhelm a server if every instance of an app hits the server around the same time. Therefore, for network requests, add some randomness to your alarms
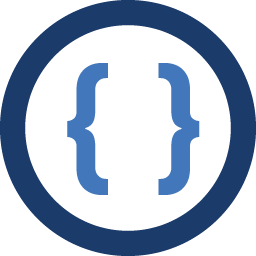
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
My program is designed to create a repeating alarm that triggers a broadcastreceiver in turn making a notification. The alarm is repeated using a user-entered interval.
For example, if i want to set the alarm to run every 10 seconds, how would I do that?
am.setRepeating(AlarmManager.RTC_WAKEUP, cal.getTimeInMillis(), 10000, calpendingintent);
Is this right? and my broadcast receiver isn't being called either for some reason.
public static void createAlarms(Context mcontext) { cal = Calendar.getInstance(); cal.add(Calendar.HOUR, alarmintervalint); calintent = new Intent(mcontext, AlarmBroadcastReceiver.class); calpendingintent = PendingIntent.getBroadcast(mcontext.getApplicationContext(), 12345, calintent, 0); am = (AlarmManager)mcontext.getSystemService(Activity.ALARM_SERVICE); am.setRepeating(AlarmManager.RTC_WAKEUP, cal.getTimeInMillis(), 10000, calpendingintent); }
My broadcastreceiver class is not being called and Im not sure the "setRepeating()" method Im using is set correctly..
Please help!
-
Admin almost 11 yearsWhat is difference between Inexact and normal? And I don't want to set the interval for a day. It is a user inputed variable (integer)
-
Admin almost 11 yearsthis is nice! thanks. but my alarm will trigger a notification so do I need to make changes?
-
Admin almost 11 yearshow can i reset this alarm? like suppose after 50 alarms, i want to reset the interval. can I just call this method? because when i try that now, its giving me a never ending loop between this method and the broadcastreceiver class.
-
Dixit Patel almost 11 yearsSimple take one Integer variable in AlarmBroadcastReceiver , when alarm triggred increase value of that variable & if variable == 50 then you have to call above stopAlarmManager() method.
-
Siddharth Sachdeva over 6 yearsIn the question asked, he is willing to set the repeated alarm, your solution will only trigger an alarm once.
-
Saeed Parand over 6 yearsdear friend , I will check this code again and edit it.
-
Siddharth Sachdeva over 6 yearsThanks, that would be better as a newly learner won't get confused by this.
-
Chanaka Fernando about 6 yearsUse setInexactRepeating() instead of setRepeating(). When you use setInexactRepeating(), Android synchronizes repeating alarms from multiple apps and fires them at the same time. This reduces the total number of times the system must wake the device, thus reducing drain on the battery. As of Android 4.4 (API Level 19), all repeating alarms are inexact. Note that while setInexactRepeating() is an improvement over setRepeating(), it can still overwhelm a server if every instance of an app hits the server around the same time. Therefore, for network requests, add some randomness to your alarms