Allow spaces in EMail validator in flutter
140
Solution 1
You don't need to use any package for validating email you can simply do it with RegExp
like below in this space is allows and then you can trim it where ever you want to use
validator: (value) {
bool emailValid = RegExp(r"^[a-zA-Z0-9.a-zA-Z0-9.!#$%&'*+-/=?^_`{|}~]+@[a-zA-Z0-9]+\.[a-zA-Z]+").hasMatch(value!);
if (value == null || value.isEmpty) {
return 'Please Enter Email Address';
}else if (emailValid == false){
return 'Please Enter Valid Email Address';
}
return null;
},
Let me know if you have any questions. Thanks
Solution 2
You can trim email first then you can check for validation.
validator: (email) {
if(email != null) {
email = email.trim();
(!EmailValidator.validate(email))
? 'Enter a valid Email' : null,
}
return null;
}
Solution 3
best practice to use TextFormField validation is to not to allow user to put irrelevant data
TextFormField(
controller: _etcEmail,
keyboardType: TextInputType.emailAddress,
textInputAction: TextInputAction.done,
inputFormatter: [
// FilteringTextInputFormatter.allow(RegExp(r"^[a-zA-Z0-9.a-zA-Z0-9.!#$%&'*+-/=?^_`{|}~]+@[a-zA-Z0-9]+\.[a-zA-Z]+")),
// FilteringTextInputFormatter.deny(RegExp(r" ")),
FilteringTextInputFormatter.allow(RegExp(r" ")),
],
hintText: 'Email',
validator: (value) {
if (value!.isEmpty) {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
backgroundColor: Colors.pinkAccent,
behavior: SnackBarBehavior.floating,
padding: EdgeInsets.all(5),
content: (Text('Email Field is Required'))));
}
},
read: false,
)
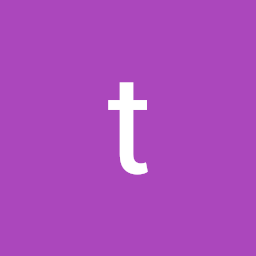
Author by
taha khamis
Updated on January 05, 2023Comments
-
taha khamis 10 months
Im using email validator package on flutter to validate the email for login. I have one issue with this package that I want to allow spaces in the email when the user sign in because Im gonna trim the text anyway so I dont want it to show error when there is Spaces at the end.
child: TextFormField( keyboardType: TextInputType.emailAddress, controller: emailController, cursorColor: Colors.white, textInputAction: TextInputAction.next, decoration: const InputDecoration(labelText: 'Email'), autovalidateMode: AutovalidateMode.onUserInteraction, validator: (email) => email != null && !EmailValidator.validate(email) ? 'Enter a valid Email' : null,
try { await _auth.signInWithEmailAndPassword( email: emailController.text.trim(), password: passwordController.text.trim(), );
Anyone knows how to do it or if there is a better way than using this package?
-
taha khamis over 1 yearThanks! I do prefer not to use any package to thank you for your suggestion
-
Randal Schwartz over 1 yearNo, please don't use this so-called "regex email validation". I can think of a few email addresses that are perfectly valid that do not match that regex. When you want to validate an email address, please do not use a hand-rolled (or even copy-n-pasted) regex. Instead, use pub.dev/packages/email_validator which properly implements the RFC822 (et. seq.) address according to the rules in the RFC. (Jokingly...) Or, cut-n-paste this one (and only this one): ex-parrot.com/~pdw/Mail-RFC822-Address.html. Don't forget to remove the newlines from that 1400-char monster. :)