Allow Web Page To Be Rendered Inside HTML Frame
Solution 1
The value of X-Frame-options can be DENY (default), SAMEORIGIN, and ALLOW-FROM uri. According to Spring Security documentation you can tell Spring to overwrite the default behaviour adding your custom header that way:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.headers()
.addHeaderWriter(new XFrameOptionsHeaderWriter(new WhiteListedAllowFromStrategy(Arrays.asList("www.yourhostname.com"))))
...
}
and Spring shall append X-Frame-Options: ALLOW-FROM ... or
.addHeaderWriter(new XFrameOptionsHeaderWriter(XFrameOptionsHeaderWriter.XFrameOptionsMode.SAMEORIGIN))
for X-Frame-Options: SAMEORIGIN or make it completely disable by
http.headers().frameOptions().disable()
Solution 2
EDIT (06.2020) - The X-Frame options are OBSOLETE:
"The frame-ancestors directive obsoletes the X-Frame-Options header. If a resource has both policies, the frame-ancestors policy SHOULD be enforced and the X-Frame-Options policy SHOULD be ignored."
https://www.w3.org/TR/CSP2/#frame-ancestors-and-frame-options
so consider using content-security-policy
:
<headers>
<content-security-policy policy-directives="frame-ancestors 'self'"/>
</headers>
If you are using Spring Security 4.x the following configuration will solve your problem (assuming the webapp runs on the same server address).
XML configuration:
<http>
<!-- ... -->
<headers>
<frame-options policy="SAMEORIGIN" />
</headers>
</http>
Java configuration:
@EnableWebSecurity
public class WebSecurityConfig extends
WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// ...
.headers().frameOptions().sameOrigin();
}
}
Disable Configuration
You could also just disable it, being aware of the security risk.
http.headers().frameOptions().disable();
Background Information
In Spring Security 3.2.0, security headers were introduced, but were disabled by default:
http://spring.io/blog/2013/08/23/spring-security-3-2-0-rc1-highlights-security-headers/
In Spring Security 4.x the headers are enabled by default (for IFrames: X-Frame-Options: DENY):
"Spring Security 4.x has changed both the Java Configuration and XML Configuration to require explicit disabling of defaults."
http://docs.spring.io/spring-security/site/migrate/current/3-to-4/html5/migrate-3-to-4-jc.html#m3to4-header
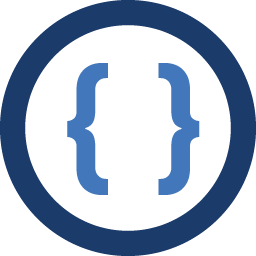
Admin
Updated on June 24, 2020Comments
-
Admin almost 4 years
I have two web applications: web application (web-app) and report web. I want to embedded report web in web-app in a
<iframe>
. So it refused by Browser with the error:X-Frame-Options: DENY
Any help?
-
Erica Kane about 8 yearsNo. Your Java listing will not compile (even though the docs say it will).
-
flavio.donze about 8 yearsWell you need to remove the "// ..." part since this is not java syntax and stands for previous configurations of the
HttpSecurity http
object. -
Erica Kane about 8 yearsI did all of that. It doesn't work. The docs are wrong, as they are in a few places with respect to the Java configuration of headers. @medveshonok117 has the right answer.
-
flavio.donze about 8 yearsWhat's wrong? You get a compile error? Configuration
configure()
doesn't get called? -
Erica Kane about 8 yearsThe problem comes in if you have multiple header options to configure. And while there are zero examples given in the documentation, I did eventually figure it out. In most cases you need to use multiple copies of
.and()
in a row. -
R.Anandan over 7 yearswhere i need to add this
-
m c over 7 yearsin your Spring Security config, e.g. as described in docs.spring.io/spring-security/site/docs/4.2.x/guides/html5 (if you are referring to v.4.2.x)