Alternative for function overloading in Go?
Solution 1
Neither function overloading nor optional arguments are directly supported. You could work around them building your own arguments struct. I mean like this (untested, may not work...) EDIT: now tested...
package main
import "fmt"
func main() {
args:=NewMyArgs("a","b") // filename is by default "c"
args.SetFileName("k")
ret := Compresser(args)
fmt.Println(ret)
}
func Compresser(args *MyArgs) string {
return args.dstFilePath + args.srcFilePath + args.fileName
}
// a struct with your arguments
type MyArgs struct
{
dstFilePath, srcFilePath, fileName string
}
// a "constructor" func that gives default values to args
func NewMyArgs(dstFilePath string, srcFilePath string) *MyArgs {
return &MyArgs{
dstFilePath: dstFilePath,
srcFilePath:srcFilePath,
fileName :"c"}
}
func (a *MyArgs) SetFileName(value string){
a.fileName=value;
}
Solution 2
The idiomatic answer to optional parameters in Go is wrapper functions:
func do(a, b, c int) {
// ...
}
func doSimply(a, b) {
do(a, b, 42)
}
Function overloading was intentionally left out, because it makes code hard(er) to read.
Solution 3
There are some hints here using variadic arguments, for example:
sm1 := Sum(1, 2, 3, 4) // = 1 + 2 + 3 + 4 = 10
sm2 := Sum(1, 2) // = 1 + 2 = 3
sm3 := Sum(7, 1, -2, 0, 18) // = 7 + 1 + -2 + 0 + 18 = 24
sm4 := Sum() // = 0
func Sum(numbers ...int) int {
n := 0
for _,number := range numbers {
n += number
}
return n
}
Or ...interface{}
for any types:
Ul("apple", 7.2, "BANANA", 5, "cHeRy")
func Ul(things ...interface{}) {
fmt.Println("<ul>")
for _,it := range things {
fmt.Printf(" <li>%v</li>\n", it)
}
fmt.Println("</ul>")
}
Related videos on Youtube
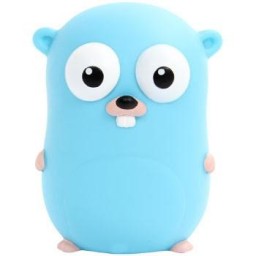
Coder
A student who is currently learning C# and Go. I am also know Chinese, Cantonese and Malays rather fluently and a little bit of Japanese. My hobby is programming and reading books that related to technology(maybe software development).
Updated on September 14, 2022Comments
-
Coder 8 months
Is it possible to work similar way like the function overloading or optional parameter in C# using Golang? Or maybe an alternative way?
-
Paolo Falabella over 10 yearscan you give a concrete example (also in c#) of what you want to do?
-
Coder over 10 years
public void Compresser(string dstFilePath, string srcFilePath, string fileName)
public void Compresser(string srcFilePath, string fileName)
-
nemo over 10 yearsPossible duplicate: stackoverflow.com/questions/2032149/optional-parameters
-
kostix over 10 yearsThat would be
func Compress(srcFilePath string, fileName string)
andfunc CompressInto(dstFilePath string, srcFilePath string, fileName string)
in Go.
-
-
Mohit Bhura over 6 yearsoptional arguments are now allowed in Go. check this out changelog.ca/log/2015/01/30/golang
-
Paolo Falabella over 6 years@MohitBhura your link is worth a read but it does not say that optional arguments are now allowed. In fact it suggest a different way to work around their absence, based on empty interfaces and variadic arguments
-
hlscalon about 6 yearsbecause it makes code hard(er) to read. That's totally subjective. I don't think so, not always.
-
Jonathan Hartley about 5 years@old_mountain I believe the Go designers agree with you that sometimes it is harder, sometimes it is easier. However, empirically, they believe that the cases where it was used badly greatly outnumbered the cases where it was used well. So overall, removing them makes code easier to read. Bear in mind the designers had decades of literally industry-defining experience.
-
Admin over 2 yearsGo Playground example play.golang.org/p/7znCwjK6zIp +1.
-
jean about 2 yearsThis is meaningless, no difference with func FlavorANewSomething()
-
Rads about 2 years@JonathanHartley You topped it with something even more subjective. "literally industry-defining" is almost a paradox. One only needs to define something that is new, and if we are talking about an existing industry, why is it being defined? Go seems to make a ton of assumptions of this sort, which is really not helpful to anyone. Poor coders will write poor code or chose a poor framework which lets them do so. Optimising for poor coders is (being subjective) a bad choice when the language tries to optimise for performance.
-
Jonathan Hartley about 2 years@Rads Yep, that's fair. Bear in mind I was replying to a comment that has since been deleted, which I'm going to speculate was outrageous.