alternative to readarray, because it does not work on mac os x
17,260
Solution 1
Here's the awk version. Note that NPars
is not hardcoded.
vars=($(awk -F= '{print $1}' varsValues.txt))
values=($(awk -F= '{print $2}' varsValues.txt))
Npars=${#vars[@]}
for ((i=0; i<$Npars; i++)); do
eval ${vars[$i]}=${values[$i]}
done
echo $people
Solution 2
You could use a read loop.
while IFS=\= read var value; do
vars+=($var)
values+=($value)
done < VarsValues.txt
Solution 3
You can use declare
bulletin:
declare -a vars=( $(cut -d '=' -f1 varsValues.txt) )
declare -a values=( $(cut -d '=' -f2 varsValues.txt) )
Solution 4
Let's start with this:
$ awk -F'=' '{values[$1]=$2} END{print values["people"]}' file
7.2
$ awk -F'=' '{values[$1]=$2} END{for (name in values) print name, values[name]}' file
languages 2014
oceans 3.4
world 1
something 20.6
countries 205
people 7.2
aa 13.7
Now - what else do you need to do?
Solution 5
perl -0777 -nE '@F= split /[=\r\n]/; say "@F[grep !($_%2), 0..$#F]"; say "@F[grep $_%2, 0..$#F]"' varsValues.txt
or by reading same file twice,
perl -F'=' -lane 'print $F[0]' varsValues.txt
perl -F'=' -lane 'print $F[1]' varsValues.txt
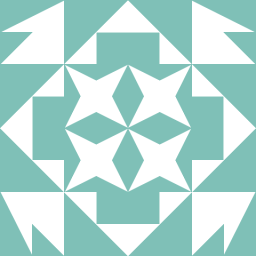
Author by
user3652962
Updated on June 16, 2022Comments
-
user3652962 11 months
I have a varsValues.txt file
cat varsValues.txt aa=13.7 something=20.6 countries=205 world=1 languages=2014 people=7.2 oceans=3.4
And I would like to create 2 arrays, vars and values. It should contain
echo ${vars[@]} aa something countries world languages people oceans echo ${values[@]} 13.7 20.6 205 1 2014 7.2 3.4
I use
Npars=7 readarray -t vars < <(cut -d '=' -f1 varsValues.txt) readarray -t values < <(cut -d '=' -f2 varsValues.txt) for (( yy=0; yy<$Npars; yy++ )); do eval ${vars[$yy]}=${values[$yy]} done echo $people 7.2
But I would like it without readarray which does not work on Mac (os x) and IFS (interfield separater).
Any other solution? awk? perl? which I can use in my bash script.
Thanks.
-
msw almost 9 yearsAnd then what? These doesn't populate shell variables as the sample script does.
-
Jotne almost 9 yearsIt should work without the
declare
, just:vars=($(cut -d '=' -f1 varsValues.txt))
work fine on my Ubuntu. -
anubhava almost 9 yearsThat's right it should work without
declare -a
also. -
chepner almost 9 years+1 This would be preferred even if
readarray
were available, as it avoids external processes and a second pass through the file. -
amc over 3 yearsIn my implementation the code works without values+=($value) to create an array, where array items can be listed with ${vars[@]}.
-
blubb about 3 yearsThis solution works perfectly, but pay attention to (the absence of) whitespace in
vars+=($var)
. Usingvars += ($var)
does not work, which surprised me a bit.