Android: Adding a fragment to an activity
The problem here is that the fragment needs a container with a view id to reside within. Giving it a layout id will result in the error you saw.
You can add the fragment to your relative layout, but to do that properly you'll need to assign it appropriate layout parameters so that it can be placed. It would be easier to just create a FrameLayout within the relative layout that wraps its contents, and then add the fragment there.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<FrameLayout
android:id="@+id/FragmentContainer"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/B1"/>
</RelativeLayout>
Then
fragmentTransaction.add(R.id.FragmentContainer, fragment);
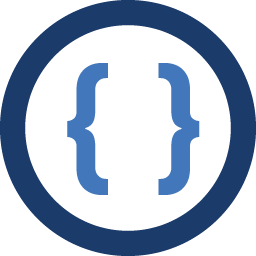
Admin
Updated on February 14, 2020Comments
-
Admin about 4 years
I'm fairly new to Android, so this might have an obvious answer, but I can't seem to find it.
I'm writing a version of a calculator app. I want to make it so that when a user clicks on one of the buttons, this starts a fragment (with more buttons on it that they can then use for more input).
The fragment code is as follows:
import android.app.Fragment; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; public class VariableMenu extends Fragment { public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState);} public void onActivityCreated(Bundle savedInstanceState) { super.onActivityCreated(savedInstanceState); } public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { return inflater.inflate(R.layout.fragment, container, false); } }
with an XML layout as follows:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <Button android:id="@+id/Bx" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="X" android:onClick="onButtonClicked"/> <Button android:id="@+id/By" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_toRightOf="@id/Bx" android:layout_alignTop="@id/Bx" android:text="Y" android:onClick="onButtonClicked"/> <Button android:id="@+id/Bz" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_toRightOf="@id/By" android:layout_alignTop="@id/By" android:text="Z" android:onClick="onButtonClicked"/> </RelativeLayout>
(I know I shouldn't be using hard-coded strings, but I'll fix that once I get the thing running)
I tried having it added using a fragmenttransaction with the onTouch method of the activating button, like so:
public void onFragmentActivated(View v) { FragmentManager fragmentManager = getFragmentManager(); FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction(); VariableMenu fragment = new VariableMenu(); fragmentTransaction.add(R.layout.main, fragment); fragmentTransaction.commit(); }
but that gave an error saying there was "No view found for id:...(main) for fragment VariableMenu."
So then I made a view for it in the main XML file:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent"> (Irrelevant things) <fragment android:id="@+id/Fragment" android:name="calculator.app.VariableMenu" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/B1"/> (Irrelevant things) </RelativeLayout>
But this resulted in the fragment being there as soon as the activity was created (and setContentView(R.layout.main); ran).
Is there a simple way to programmatically add and remove a fragment with a UI to an activity?