Android - Caused by: java.security.cert.CertPathValidatorException: Trust anchor for certification path not found
First of all you need to install the self-signed SSL .cer file in the device Security Settings. Then you need to add a network_security_config.xml and add it in your manifest.
android:networkSecurityConfig="@xml/network_security_config"
There are several ways to configure the network security:ways to trust certificates. I think the best way is trusting custom CAs only for debugging:
<network-security-config>
<debug-overrides>
<trust-anchors>
<!-- Trust user added CAs while debuggable only -->
<certificates src="user" />
</trust-anchors>
</debug-overrides>
But you can also just always trust user added CAs:
<network-security-config>
<base-config>
<trust-anchors>
<certificates src="system"/>
<certificates src="user"/>
</trust-anchors>
</base-config>
Or you can specify wich certificates are trusted, here the marvelous description
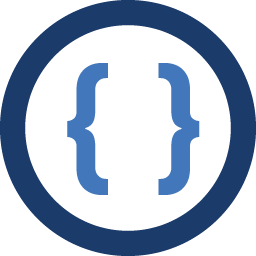
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm using self-signed CA certificate for testing environment but facing issue to hit api using HttpClient.... Please help me to fix this issue....
W/System.err: javax.net.ssl.SSLHandshakeException: java.security.cert.CertPathValidatorException: Trust anchor for certification path not found. / W/System.err: at com.android.org.conscrypt.OpenSSLSocketImpl.startHandshake(OpenSSLSocketImpl.java:333) at com.android.okhttp.internal.http.SocketConnector.connectTls(SocketConnector.java:103) at com.android.okhttp.Connection.connect(Connection.java:167) at com.android.okhttp.Connection.connectAndSetOwner(Connection.java:209) at com.android.okhttp.OkHttpClient$1.connectAndSetOwner(OkHttpClient.java:128) at com.android.okhttp.internal.http.HttpEngine.nextConnection(HttpEngine.java:352) at com.android.okhttp.internal.http.HttpEngine.connect(HttpEngine.java:341) at com.android.okhttp.internal.http.HttpEngine.sendRequest(HttpEngine.java:259) at com.android.okhttp.internal.huc.HttpURLConnectionImpl.execute(HttpURLConnectionImpl.java:454) at com.android.okhttp.internal.huc.HttpURLConnectionImpl.connect(HttpURLConnectionImpl.java:114) at com.android.okhttp.internal.huc.HttpURLConnectionImpl.getOutputStream(HttpURLConnectionImpl.java:245) at com.android.okhttp.internal.huc.DelegatingHttpsURLConnection.getOutputStream(DelegatingHttpsURLConnection.java:218) at com.android.okhttp.internal.huc.HttpsURLConnectionImpl.getOutputStream(HttpsURLConnectionImpl.java) at .Components.HttpClient.getInternetData(HttpClient.java:135) at .Components.HttpClient.doInBackground(HttpClient.java:53) at .Components.HttpClient.doInBackground(HttpClient.java:31) 03-11 20:18:19.305 8156-8238/ W/System.err: at android.os.AsyncTask$2.call(AsyncTask.java:295) at java.util.concurrent.FutureTask.run(FutureTask.java:237) at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1113) at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:588) at java.lang.Thread.run(Thread.java:818) Caused by: java.security.cert.CertificateException: java.security.cert.CertPathValidatorException: Trust anchor for certification path not found. at com.android.org.conscrypt.TrustManagerImpl.checkTrusted(TrustManagerImpl.java:324) at com.android.org.conscrypt.TrustManagerImpl.checkServerTrusted(TrustManagerImpl.java:225) at com.android.org.conscrypt.Platform.checkServerTrusted(Platform.java:115) at com.android.org.conscrypt.OpenSSLSocketImpl.verifyCertificateChain(OpenSSLSocketImpl.java:571) at com.android.org.conscrypt.NativeCrypto.SSL_do_handshake(Native Method) 03-11 20:18:19.306 8156-8238/ W/System.err: at com.android.org.conscrypt.OpenSSLSocketImpl.startHandshake(OpenSSLSocketImpl.java:329) ... 20 more
Here is my calling method:
HttpClient client = new HttpClient(context, new ReturnProcess(), params_hash_map); client.executeOnExecutor(AsyncTask.THREAD_POOL_EXECUTOR, URL);
Base Class:
import android.content.Context; import android.net.ConnectivityManager; import android.net.NetworkInfo; import android.os.AsyncTask; import android.widget.Toast; import org.apache.http.client.ClientProtocolException; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.io.OutputStreamWriter; import java.net.HttpURLConnection; import java.net.URI; import java.net.URL; import java.util.HashMap; import java.util.Iterator; import java.util.LinkedHashMap; import javax.net.ssl.HttpsURLConnection; import javax.net.ssl.SSLContext; import javax.net.ssl.SSLSocketFactory; public class HttpClient extends AsyncTask<String, String, String> { Context context; CallReturn callReturn; LinkedHashMap params; public HttpClient (Context context, CallReturn callReturn, LinkedHashMap params) { this.context = context; this.callReturn = callReturn; this.params = params; } @Override protected String doInBackground(String... url) { String responseString = null; try { if (isNetworkConnected()) { responseString = getInternetData(url[0], params); if (responseString.equalsIgnoreCase("ENDUP") || responseString.equalsIgnoreCase("logout")) { // Logs.isLogout = true; } } else { responseString = "NoInterNet"; } } catch (ClientProtocolException e) { responseString = "ClientProtocolException"; Utilities.handleException(e); } catch (IOException e) { responseString = "IOException"; Utilities.handleException(e); } catch (Exception e) { responseString = "Exception"; Utilities.handleException(e); } return responseString; } @Override protected void onPostExecute(String s) { super.onPostExecute(s); callReturn.onCallCompleted(s); } public boolean isNetworkConnected() { ConnectivityManager cm = (ConnectivityManager) context.getSystemService(Context.CONNECTIVITY_SERVICE); NetworkInfo ni = cm.getActiveNetworkInfo(); if (ni == null) { return false; // There are no active networks. } else { return true; } } private String getInternetData(String uri, LinkedHashMap params) throws Exception { // BufferedReader in = null; String data = null; try { /* NEW Working */ // String url = params[0]; String USER_AGENT = "Mozilla/5.0"; StringBuilder tokenUri = new StringBuilder(); Iterator iterator = params.keySet().iterator(); while (iterator.hasNext()) { String key=(String)iterator.next(); String value=(String)params.get(key); tokenUri.append(key + value); } URL obj = new URL(uri); HttpURLConnection con = (HttpURLConnection) obj.openConnection(); con.setRequestMethod("POST"); con.setRequestProperty("User-Agent", USER_AGENT); con.setRequestProperty("Accept-Language", "UTF-8"); con.setDoOutput(true); OutputStreamWriter outputStreamWriter = new OutputStreamWriter(con.getOutputStream()); outputStreamWriter.write(tokenUri.toString()); outputStreamWriter.flush(); BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream())); String inputLine; StringBuilder response = new StringBuilder(); while ((inputLine = in.readLine()) != null) { response.append(inputLine); } in.close(); data = response.toString(); return data; } catch (Exception ex) { Utilities.handleException(ex); return data; } // finally // { // if (in != null) // { // try // { // in.close(); // return data; // } // catch (Exception e) // { // Utilities.handleException(e); // } // } // } } }