Android Color Notification Icon
Solution 1
I found the answer to my question here: https://stackoverflow.com/a/44950197/4394594
I don't know entirely what the problem was, but by putting the huge png that I was using for the icon into the this tool https://romannurik.github.io/AndroidAssetStudio/icons-notification.html#source.type=image&source.space.trim=1&source.space.pad=0&name=ic_skylight_notification
and by placing the generated icons it gave into my mipmap folder, I was able to get the setColor(...)
property to work correctly.
Solution 2
For firebase nofitications sent from console you just need to add this in your manifest:
<meta-data
android:name="com.google.firebase.messaging.default_notification_icon"
android:resource="@drawable/white_logo" />
<meta-data
android:name="com.google.firebase.messaging.default_notification_color"
android:resource="@color/custom_color" />
Where white_logo is your app white logo, and custom_color is the color you want to have the icon and text colored.
More details here: https://firebase.google.com/docs/cloud-messaging/android/client
Solution 3
Here is what I did for my app ...
private void showNotification(Context context) {
Log.d(MainActivity.APP_TAG, "Displaying Notification");
Intent activityIntent = new Intent(context, MainActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, activityIntent, PendingIntent.FLAG_UPDATE_CURRENT);
NotificationCompat.Builder mBuilder = new NotificationCompat.Builder(context);
mBuilder.setSmallIcon(R.drawable.ic_notification);
mBuilder.setColor(Color.GREEN);
mBuilder.setContentIntent(pendingIntent);
mBuilder.setContentTitle("EarthQuakeAlert");
mBuilder.setContentText("It's been a while you have checked out earthquake data!");
mBuilder.setDefaults(Notification.DEFAULT_SOUND);
mBuilder.setAutoCancel(true);
NotificationManager mNotificationManager = (NotificationManager) context.getSystemService(Context.NOTIFICATION_SERVICE);
mNotificationManager.notify(1, mBuilder.build());
}
Sample With Color:
Solution 4
When building the notification, you can set the color and the icon. If your icon is a pure white image, it'll apply the color for you in the correct spots.
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
val manager = context.getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager
val notificationId = 10 // Some unique id.
// Creating a channel - required for O's notifications.
val channel = NotificationChannel("my_channel_01",
"Channel human readable title",
NotificationManager.IMPORTANCE_DEFAULT)
manager.createNotificationChannel(channel)
// Building the notification.
val builder = Notification.Builder(context, channel.id)
builder.setContentTitle("Warning!")
builder.setContentText("This is a bad notification!")
builder.setSmallIcon(R.drawable.skull)
builder.setColor(ContextCompat.getColor(context, R.color.colorPrimary))
builder.setChannelId(channel.id)
// Posting the notification.
manager.notify(notificationId, builder.build())
}
Solution 5
I might be late to the party but all above answers are not relevant or deprecated.
You can achieve this easily by using setColor
method of NotificationCompat.Builder
Example:
val builder = NotificationCompat.Builder(this, "whatever_channel_id")
.setSmallIcon(R.drawable.ic_notification) //set icon for notification
.setColor(ContextCompat.getColor(this, R.color.pink))
.setContentTitle("Notification Title")
.setContentText("Notification Message!")
Now it will show notification as pink color
Note: If you are using firebase then the color won't be seen directly. You have to add this in manifest file.
<meta-data
android:name="com.google.firebase.messaging.default_notification_icon"
android:resource="@drawable/ic_notification" />
<meta-data
android:name="com.google.firebase.messaging.default_notification_color"
android:resource="@color/pink" />
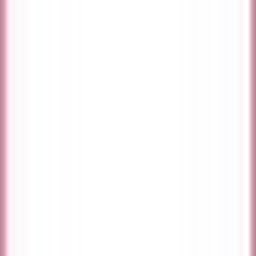
Oblivionkey3
Updated on July 08, 2022Comments
-
Oblivionkey3 almost 2 years
I'm working on an app where I create a notification for the user. I want the icon to appear as white when it's in the status bar, but colored blue when it's being displayed in the drop down notification menu. Here's an example of the same thing being done by the Google Store app.
White notification in status bar:
Colored notification in drop down menu:
How can I replicate this? What properties do I have to set?
Edit: Here's my current code - I made the image all white with a transparent background, so it looks fine in the status bar, but in the notification drop, the image is still the same white color:
private NotificationCompat.Builder getNotificationBuilder() { return new NotificationCompat.Builder(mainActivity) .setDeleteIntent(deletedPendingIntent) .setContentIntent(startChatPendingIntent) .setAutoCancel(true) .setSmallIcon(R.drawable.skylight_notification) .setColor(ContextCompat.getColor(mainActivity, R.color.colorPrimary)) .setContentTitle(mainActivity.getString(R.string.notification_title)) .setContentText(mainActivity.getString(R.string.notification_prompt)); }
-
Oblivionkey3 almost 7 yearsUnfortunately all that changing the color did for me was change the color of the text next to the image in the notification drop down, not change the color of the image at all.
-
advice almost 7 yearsAre you using a pure white icon as your small icon? It should colour that when it needs to.
-
Oblivionkey3 almost 7 yearsIt's pure white with a transparent background, and it's saved as a png
-
advice almost 7 yearsI edited my post, and tested some code on O, and the code above works for making my icon and text both my
color_primary
when pulled down, and just pure white when not. Hopefully that'll help more. -
advice almost 7 yearsI'd advise against this or else you'll have a coloured icon within the status bar.
-
Oblivionkey3 almost 7 yearsI appreciate your help, but I managed to find solve this using another method which I just added to this page.
-
advice almost 7 yearsCool, glad you could figure it out.
-
Andrew Leader over 6 yearsTHANK YOU! I spent way too long trying to figure out why my notification icon was still appearing white in the notification banner, and finally with that tool my notification icon correctly uses the color in the banner (while still being white in the status bar)
-
Mikolaj Kieres almost 6 yearsTo everyone who ended up here and is genering icons with photoshop (or any other graphic design tool). Don't use CMYK mode! Use RGB when creating/exporting an icon - it helped in my case
-
Pradip Tilala about 5 yearsAdded the icon as suggested by the "Oblivionkey3" but after
adding <meta-data android:name="com.google.firebase.messaging.default_notification_color" android:resource="@color/custom_color" />
icon color set to custom. Before this icon color was grey on Andriod Oreo notification (Status bar was icon being displayed in white). -
mukesh.kumar almost 5 yearswhat is
custom_color
? and how to set it? -
Raul Pinto over 4 years
-
radu_paun over 4 years@mukesh.kumar, custom_color is any color you want to use. you just need to declare it in colors file
-
Michael Alan Huff over 3 yearsGod bless you Advice-Dog. This was the answer I needed tonight.
-
Bip901 over 3 yearsI used this tool, my image is a pure white transparent PNG and I made sure it's in the RGB color space, but
SetColor
still doesn't work. What am I missing? -
Bip901 over 3 yearsTo anyone still stuck, try downscaling the image. A 72x72 icon didn't work for me, but 48x48 did.
-
Johann over 2 yearsThey should have called "setColor" "setIconColor" instead and it woud have been obvious.