Android column '_id' does not exist?
Solution 1
I see, the documentation for CursorAdapter states:
The Cursor must include a column named
_id
or this class will not work.
The SimpleCursorAdapter
is a derived class, so it appears this statement applies. However, the statement is technically wrong and somewhat misleading to a newbie. The result set for the cursor must contain _id
, not the cursor itself.
I'm sure this is clear to a DBA because that sort of shorthand documentation is clear to them, but for those newbies, being incomplete in the statement causes confusion. Cursors are like iterators or pointers, they contain nothing but a mechanism for transversing the data, they contain no columns themselves.
The Loaders documentation contains an example where it can be seen that the _id
is included in the projection parameter.
static final String[] CONTACTS_SUMMARY_PROJECTION = new String[] {
Contacts._ID,
Contacts.DISPLAY_NAME,
Contacts.CONTACT_STATUS,
Contacts.CONTACT_PRESENCE,
Contacts.PHOTO_ID,
Contacts.LOOKUP_KEY,
};
public Loader<Cursor> onCreateLoader(int id, Bundle args) {
// ...
return new CursorLoader(getActivity(), baseUri,
CONTACTS_SUMMARY_PROJECTION, select, null,
Contacts.DISPLAY_NAME + " COLLATE LOCALIZED ASC");
}
Solution 2
This has been answered and I would like to make it more comprehensive here.
SimpleCursorAdapter requires that the Cursor's result set must include a column named exactly "_id". Don't haste to change schema if you didn't define the "_id" column in your table. SQLite automatically added an hidden column called "rowid" for every table. All you need to do is that just select rowid explicitly and alias it as '_id' Ex.
SQLiteDatabase db = mHelper.getReadableDatabase();
Cursor cur = db.rawQuery( "select rowid _id,* from your_table", null);
Solution 3
Tim Wu's code really works...
If you are using db.query, then it would be like this...
db.query(TABLE_USER, new String[] {
"rowid _id",
FIELD_USERNAME,
},
FIELD_USERNAME + "=" + name,
null,
null,
null,
null);
Solution 4
Yes , I also change the SELECT string query to fix this issue.
String query = "SELECT t.*,t.id as _id FROM table t ";
Solution 5
What solved my issue with this error was that I had not included the _id column in my DB query. Adding that solved my problem.
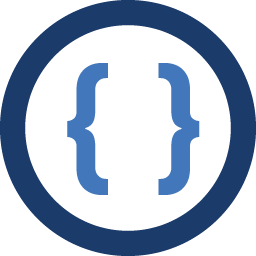
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I'm having trouble with something that works in the Notepad example. Here's the code from the NotepadCodeLab/Notepadv1Solution:
String[] from = new String[] { NotesDbAdapter.KEY_TITLE }; int[] to = new int[] { R.id.text1 }; SimpleCursorAdapter notes = new SimpleCursorAdapter(this, R.layout.notes_row, c, from, to);
This code seems to work fine. But just to be clear, I ran the ADB utility and run SQLite 3. I inspected the schema as follows:
sqlite> .schema
CREATE TABLE android_metadata (locale TEXT); CREATE TABLE notes (_id integer primary key autoincrement, title text not null, body text not null);
All seems good to me.
Now on to my application, which, as far as I can see, is basically the same with a few minor changes. I've simplified and simplified my code, but the problem persists.
String[] from = new String[] { "x" }; int[] to = new int[] { R.id.x }; SimpleCursorAdapter adapter = null; try { adapter = new SimpleCursorAdapter(this, R.layout.circle_row, cursor, from, to); } catch (RuntimeException e) { Log.e("Circle", e.toString(), e); }
When I run my application, I get a RuntimeException and the following prints in LogCat from my
Log.e()
statement:LogCat Message:
java.lang.IllegalArgumentException: column '_id' does not exist
So, back to SQLite 3 to see what's different about my schema:
sqlite> .schema CREATE TABLE android_metadata (locale TEXT); CREATE TABLE circles (_id integer primary key autoincrement, sequence integer, radius real, x real, y real);
I don't see how I'm missing the '_id'.
What have I done wrong?
One thing that's different between my application and the Notepad example is that I started by creating my application from scratch using the Eclipse wizard while the sample application comes already put together. Is there some sort of environmental change I need to make for a new application to use a SQLite database?
-
user405821 almost 14 yearsI recommend the statement in the documentation be written as "The Cursor's result set must include a column named exactly "_id". Failing to do so will produce a RuntimeException." This would be less confusing and correct.
-
tpow over 13 years@user405821 - Thanks man - this guy didn't mark your answer as correct - but this was an excellent addition to a part of Android that is poorly documented. +1
-
Ben H over 12 yearsi made a note about in the android docs using google sidewiki. sidewiki is perfect for this sort of thing, and i hope that more people use it in conjunction with the android documentation. developer.android.com/reference/android/widget/…
-
fikr4n almost 12 yearsHow about overriding
getItemId
and provide my own defined ID? Can it solve the problem? -
tom_mai78101 almost 11 yearsThis is when all solutions fail to fix the problem. The last resort to everything.
-
the_dark_destructor almost 11 yearsOh man, this is the amazing Android SDK + documentation for you! Thank you so much for the answer. I spent more than an hour trying to figure out what the problem was.
-
Mike Baxter almost 11 years@max4ever has the best answer. It is the perfect solution to this issue. No need to start creating unnecessary _id columns in your database
-
Admin over 10 yearsThis is an easy mistake to make; the official documentation says to use _ID.
-
Vish over 8 yearsThanks! In my case the error was due to a problem of uppercase v/s lowercase.
-
Stan about 8 years+1, Using "rowid _id" in a query really helps. But what a mess is Android if its default DB implementation creates
rowid
key while its default cursor adapter requires_id
?! -
TryinHard over 7 yearsWorked for me too. This is the answer that saves me from adding _id into my database.
-
Shark Deng over 4 yearsThanks! I change database eid to _id, uninstalled previous one and reinstall the app. Now it works.