Android Firebase setValue() Permission Denied
Solution 1
I was too stuck on this point and here's what helped me.
First things first, there are two types of users who can access database from firebase
- Authorized
- Non-authorized
By default it is set to non-authorized but then they do not have any permissions neither read nor write, so initially if you try to perform any operation you get the permission denied error.
How to change this to suit your requirement?
Solution:
Under Database section, go to Rules tab. The URL will be something like this with your project name.
https://console.firebase.google.com/project/project-name/database/rules
You will see somthing like this here:
{
"rules": {
".read": "auth != null", //non-authorised users CANT read
".write": "auth != null" //non-authorised users CANT write
}
}
Just change this to
{
"rules": {
".read": "auth == null", //even non-authorised users CAN read
".write": "auth == null" //even non-authorised users CAN write
}
}
Change this as per your requirement. Happy coding :)
Solution 2
I had the wrong path in my DB Reference.
http://DB-PATH/simplelogin:00/PERMISSION-DENIED
http://DB-PATH/users/simplelogin:00/Can-only-add-here
Solution 3
For people who have this problem, do not forget to change the "type of database" that by default gives us firebase, it should be noted that there is 2 type of database one that is Cloud Firestore and another that is RealTime Database
To answer the question, make sure you are using Realtime database:
Then edit the rules:
Obviously this configuration of rules should only be used for development environments, do not forget to change the rules when it is in production.
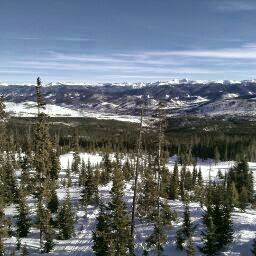
thurst0n
If you'd like to contact me you can do so by emailing me at [email protected] Thank you.
Updated on June 17, 2022Comments
-
thurst0n almost 2 years
This is how the rules are defined on firebase:
{ "rules": { "users": { ".read": true, "$user_id": { ".write": "auth.uid === $user_id", ".read" : true }}}}
I have succesfully written new user information in my register activity using setValue() just like below, and also added new comments using push().setValue(), each time I didn't have an issue with authentication. New user written straight to http://DB/users/ while comments were added very deep.
Here is a code snippit, my code hits the Log: Data could not be saved. Everytime.
AuthData authData = newRef.getAuth(); if (authData != null) { Log.v(LOG_TAG, "Auth as " + authData.getUid()); Map<String, Object> newEntry = new HashMap<String, Object>(); newEntry.put("Name", name); newEntry.put("Description", desc); newEntry.put("DueDate", date); newEntry.put("Status", 0); newRef.setValue(newEntry, new Firebase.CompletionListener() { @Override public void onComplete(FirebaseError firebaseError, Firebase firebase) { if (firebaseError != null) { Log.v(LOG_TAG, "Data could not be saved. " + firebaseError.getMessage()); //Hits here every time. } else { Log.v(LOG_TAG, "Data saved successfully. Finishing activity..."); finish(); } } }); ; } else{ Log.v(LOG_TAG, "No authdata"); }
Hopefully I've provided enough information, I'm guessing the issue is in the security/rules but any direction/guidance would be very appreciated. Thank you.
-
Willis about 9 yearsWhat version of Firebase are you using. Here is a post that describes a "Permission Denied" error being thrown when the
setValue()
method was called under certain conditions: stackoverflow.com/questions/19817709/…. According to the accepted answer this was fixed as of version 1.0.10 -
thurst0n about 9 yearsI have the following in my dependencies: compile 'com.firebase:firebase-client-android:2.2.3+'
-
thurst0n about 9 yearsJust want to add, I tried updateChildren() instead of setValue() just as an alternative and it returns the same Permission Denied.
-
thurst0n about 9 yearsUghgh, sorry to anyone who wasted their time, my URL path was incorrect. I feel like a complete idiot.
-
Frank van Puffelen about 9 yearsThanks for letting us know about the fix @thurst0n. I'll vote to close, based on it being a typo.
-
thurst0n about 9 yearsFair enough! I was starring at that path forever but I wasn't checking the beginning just the end. heh.
-
-
Abdul Waheed over 5 yearsWhen I opened database rules I found service cloud.firestore { match /databases/{database}/documents { match /{document=**} { allow read, write: if true; } } } but if I am adding your mentioned code...fire base is not recognizing... any help