Android - Hide all shown Toast Messages
Solution 1
My solution was to initialize a single Toast in the activity. Then changing its text on each click.
Toast mToast = Toast.makeText(this, "", Toast.LENGTH_SHORT);
if (a) {
mToast.setText("This is a");
mToast.show();
} else if (b) {
mToast.setText("This is b");
mToast.show();
}
Solution 2
how do I disable all toast messages being process currently?
You can cancel individual Toasts
by calling cancel()
on the Toast
object. AFAIK, there is no way for you to cancel all outstanding Toasts
, though.
Solution 3
What about checking if a toast is already being displayed?
private Toast toast;
...
void showToast() {
if (toast == null || toast.getView().getWindowVisibility() != View.VISIBLE) {
toast = Toast.makeText(getActivity(), "Toast!", Toast.LENGTH_LONG);
toast.show();
}
}
Solution 4
Mudar's solution worked beautifully for me on a similar problem - I had various toasts stacking up in a backlog after multiple button
clicks.
One instance of Toast with different setText()s
and show()
s was the exactly the answer I was looking for - previous message cancelled as soon as a new button is clicked. Spot on
Just for reference, here's what I did...
In OnCreate
:
final Toast myToast = Toast.makeText(getBaseContext(), "", Toast.LENGTH_SHORT);
Within in each OnClick
:
myToast.setText(R.string.toast1);
myToast.show();
Solution 5
My solution is to save all toast references in a list and make a method to cancel all them when need it:
private ArrayList<Toast> msjsToast = new ArrayList<Toast>();
private void killAllToast(){
for(Toast t:msjsToast){
if(t!=null) {
t.cancel();
}
}
msjsToast.clear();
}
When you create a Toast do this way and save the reference:
Toast t = Toast.makeText(context, "Download error: xx", Toast.LENGTH_LONG);
t.show();
msjsToast.addToast(t);
When you need to delete them:
killAllToast();
You can create this like a static method in a global class and use it to kill all the toast of the app.
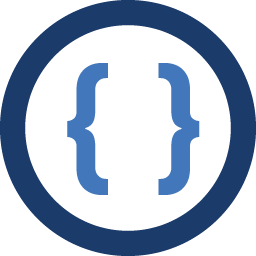
Admin
Updated on August 12, 2020Comments
-
Admin over 3 years
How do I remove all toast messages currently displayed?
In my App, there is a list, when a user clicks on an item, a toast message is displayed, 10 items - 10 toast messages.
So if the user clicks 10 times, then presses the menu button, they have to wait for some seconds until they're able to read the menu option text.
It shouldn't be like that :)
-
Admin almost 14 yearshmm, thanks a lot, will try to create something. but if i add all toast messages to some kind of structure... is there a possibility to check which ones have been shown and which not. I'm kind of busy meanwhile... sry to ask and not to try, at least i'll respond with trying to build something (at the latest) at the end of the week. -thanks!-
-
Cody Caughlan over 13 yearsThis is my latest Android pet peeve: no ability to cancel all queued Toasts.
-
cV2 over 13 yearsthanks for your answer, CommonsWare above already explained to disable a list of queued toast messages... :) thx, but sry.. i think u misunderstood the question!
-
Simon Forsberg almost 11 yearsAlthough this is a good answer, indeed it is not actually the same behavior. With this solution, you can't ever have two toasts that are showing after each other.
-
Simon Forsberg almost 11 yearsI'm downvoting this simply because I feel that it doesn't add anything new. It could have been a comment to Mudar's answer instead.
-
Simon Forsberg almost 11 yearsThis is basically the same as Mudar's answer.
-
Simon Forsberg almost 11 yearsYour Handler.postDelayed solution is interesting, +1 for that.
-
Simon Forsberg almost 11 yearsThat doesn't disables them, that just never shows them. Not the same thing!
-
Scott Solmer almost 10 yearsWelcome to SO & Thanks for sharing. Try to add some descriptive text to your code-only answer. "How do I write a good answer?". Brevity is acceptable, but fuller explanations are better.
-
Pavel Alexeev over 9 yearsWhat a disappointment: if you cancel the current toast, subsequent toasts will be displayed only after amount of time this toast was about to show.
-
pete over 6 yearsThis worked best for me as I had a pair of Sliders that could each generate several Toasts per second when the user was playing with them. Upvoting with thanks for your contribution.
-
Saman Sattari about 5 yearsYes This is something like an android joke :D
-
Noor Hossain over 3 yearsI have used your approach