Android how take full screen with more LinearLayout orientation horizontal
Using Layout Weight is a little bit tricky task. Suppose you want to show three buttons horizontally which share equal width in a linear layout. Then the xml code should be like this.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:weightSum="3"
android:orientation="horizontal" >
<Button
android:layout_height="wrap_content"
android:layout_width="0dp"
android:layout_weight="1"
android:text="Button 1"
/>
<Button
android:layout_height="wrap_content"
android:layout_width="0dp"
android:layout_weight="1"
android:text="Button 1"
/>
<Button
android:layout_height="wrap_content"
android:layout_width="0dp"
android:layout_weight="1"
android:text="Button 1"
/>
</LinearLayout>
As you can see,In the parent LinearLayout i used weightSum as 3 and in each child button i set the width to 0dp and weight to 1 and there are three buttons so it will share equal width.
By the way the root parent (RelativeLayout) is not closed in your given layout.
EDIT:2
As you said here is the code divide each LinearLayout equally with layout_weight
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:weightSum="3"
android:orientation="vertical" >
<LinearLayout
android:layout_height="0dp"
android:layout_weight="1"
android:layout_width="match_parent"
android:background="#00FF00"
>
</LinearLayout>
<LinearLayout
android:layout_height="0dp"
android:layout_weight="1"
android:layout_width="match_parent"
android:background="#FF0000"
>
</LinearLayout>
<LinearLayout
android:layout_height="0dp"
android:layout_weight="1"
android:layout_width="match_parent"
android:background="#0000FF"
>
</LinearLayout>
</LinearLayout>
OUTPUT :
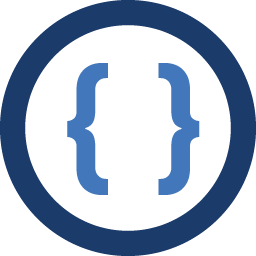
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
i'm trying to do a school day and i use multiple linearLayout with days and hours. With the
layout_weight
i can set the space for every elements inside the singleLinearLayout
. But my problem is how can i set thelayout_weight
for theLinearLayout
because they are set with orientation horizontal and they don't take the entire screen. It's like a table but i use LinearLayout because i need to create only rows.This is the xml of the Activity
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context="com.ddz.diarioscolastico.OrarioActivityProva"> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_weight="1" android:layout_alignParentTop="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/linearLayout"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginLeft="20dp" android:layout_weight="2" android:gravity="center" android:textAppearance="?android:attr/textAppearanceMedium" android:text="Lun" android:id="@+id/textView2" /> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="2" android:gravity="center" android:textAppearance="?android:attr/textAppearanceMedium" android:text="Mar" android:id="@+id/textView" /> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="2" android:gravity="center" android:textAppearance="?android:attr/textAppearanceMedium" android:text="Mer" android:id="@+id/textView3" /> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="2" android:gravity="center" android:textAppearance="?android:attr/textAppearanceMedium" android:text="Gio" android:id="@+id/textView4" /> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="2" android:gravity="center" android:textAppearance="?android:attr/textAppearanceMedium" android:text="Ven" android:id="@+id/textView5" /> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="2" android:gravity="center" android:textAppearance="?android:attr/textAppearanceMedium" android:text="Sab" android:id="@+id/textView6" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_weight="1" android:layout_below="@+id/linearLayout" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/linearLayout2"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="1" android:id="@+id/textView7" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@+id/linearLayout2" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/linearLayout3"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="2" android:id="@+id/textView8" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@+id/linearLayout3" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/linearLayout4"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="3" android:id="@+id/textView9" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@+id/linearLayout4" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/linearLayout6"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="4" android:id="@+id/textView10" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@+id/linearLayout6" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/linearLayout5"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="5" android:id="@+id/textView11" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@+id/linearLayout5" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/linearLayout7"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="6" android:id="@+id/textView12" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@+id/linearLayout7" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/linearLayout8"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="7" android:id="@+id/textView13" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@+id/linearLayout8" android:layout_alignParentLeft="true" android:layout_alignParentStart="true"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="8" android:id="@+id/textView14" /> </LinearLayout>
As you can see if i set the
android:layout_height="match_parent"
yes allLinearLayout
take full screen but only the first is visible, i wanna divide the screen for all. -
Admin about 9 yearsI forget to copy it! Thanks for your answere, but i need to divide the screen for all LinearLayout vertically. If i put all the LinearLayout inside another LinearLayout i can set the weight of the others.
-
theapache64 about 9 yearsAbsolutely you could be able to set the child LinearLayout with weight.
-
Admin about 9 yearsYes, it's exacly what i mean and what i need. Thank you!