Android / Java convert String date to long type
31,167
Solution 1
You can use the following code to get a long value (milliseconds since January 1, 1970, 00:00:00 GMT) from a String date with the format "dd/mm/yyyy".
try {
String dateString = "30/09/2014";
SimpleDateFormat sdf = new SimpleDateFormat("dd/MM/yyyy");
Date date = sdf.parse(dateString);
long startDate = date.getTime();
} catch (ParseException e) {
e.printStackTrace();
}
Solution 2
Use like this your code
String[] dateArray = dateString.split("-");
int year = Integer.parseInt(dateArray[0]);
int month = Integer.parseInt(dateArray[1]);
int date = Integer.parseInt(dateArray[2]);
GregorianCalendar gc = new GregorianCalendar(year,month,date);
long timeStamp = gc.getTimeInMillies();
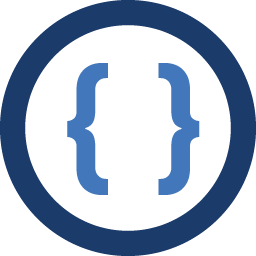
Author by
Admin
Updated on March 31, 2020Comments
-
Admin about 4 years
I need to convert a string in the format "dd/mm/yyyy", to a long type. In order to pass the value to the calendarProvider in android.
Currently I have:
Calendar calendar = Calendar.getInstance(); long startEndDate = 0; Calendar currentDateInfo = Calendar.getInstance(); currentDateInfo.set(calendar.get(Calendar.YEAR), calendar.SEPTEMBER, calendar.get(Calendar.DAY_OF_MONTH)); startEndDate = currentDateInfo.getTimeInMillis();
I need:
long startDate = *Some sort of conversion* EditText.getText();
I've tried using SimpleDateFormat but i'm having problems getting the correct type back. Any help would be much appreciated.