Android java.lang.NoClassDefFoundError: org.jsoup.Jsoup
Solution 1
I encountered this exact problem after a recent update of the ADT component of Android. It looks like Android is ignoring the build path and instead using the ANT defaults.
I solved this by removing my jar file from the build path, creating a folder in the "root" of the app heirarchy (alongside src, gen, etc) called "libs", and putting my .jar there. When you clean / build and re-launch the app, the error should go away.
FYI - this is occurring because the JAR file is not getting packaged into the .apk files - this is why it builds correctly, but isn't available during run-time on your Android device.
see NoClassDefFoundError - Eclipse and Android
Solution 2
There's also the issue that jars have to be in the libs (with an 's') directory and not lib....could this be your problem?
Solution 3
You don't need the line:
<uses-library android:name = "org.jsoup.Jsoup"/>
in your manifest file. All you need to do to use Jsoup is to ensure it's part of your build path by doing the following:
- Right click on your project and select Properties
- Select Java Build Path and select Add External JARs...
- Navigate to the Jsoup jar file
Then, Jsoup will act like any other library in java. For example in my app I use it like so:
try
{
Document doc = Jsoup.connect( myHtml ).get();
Elements table = doc.select( "table#ctl00_ContentPlaceHolder1_tblPasses" );
// Returns first row of the table which we want
Elements row = table.select( "tr.lightrow" );
Element record_One = row.first();
}
Note - I have not included all my code for it because it's rather long. Anyway, after that you just import the classes as needed, for example in mine I use the following:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
Solution 4
- Right click on the project name > Properties > Java Build Path > tab Libraries then click on button Add External jars.
- select jar's path from your directory where you had downloaded jsoup-1.7.3.jar.
- After adding jar go to next tab Order and export and select checkbox jsoup-1.7.3.jar 4 click ok 5.clean build your project and then run.
I solved the same problem by following above steps
Solution 5
You are doing it wrong. You cant use Jsoup functions in the oncreate of Activity. You need to make either Async Task or thread.
This is to prevent doing blocking tasks in you activity.
Here is a nice blog that explain how to do it and provide sample code as well.
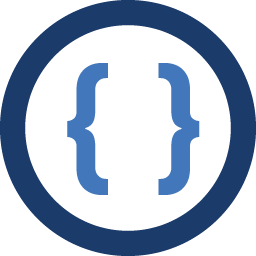
Admin
Updated on July 27, 2022Comments
-
Admin almost 2 years
I work with eclipse Version: Indigo Service Release 2 Build id: 20120216-1857. The Android version ist 2.2. I make an app to test a connect and parse a web site like this:
public class TestActivity extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); try { Document doc = Jsoup.connect("http://example.com/").get(); Elements divs = doc.select("div#test"); for (Element div : divs) { System.out.println(div.text()); } } catch (Exception e) { } } }
Manifest file:
android:installLocation="preferExternal" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" /> <uses-permission android:name="android.permission.INTERNET" /> <application android:icon="@drawable/ic_launcher" android:label="@string/app_name" > <activity android:name=".TestActivity" android:label="@string/app_name" android:configChanges="orientation" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> <uses-library android :name="org.jsoup.Jsoup" /> </activity> </application>
I add the library jsoup-1.6.1.jar to JAVA Buildpath, but I become the runtime error: E/AndroidRuntime(736): java.lang.NoClassDefFoundError: org.jsoup.Jsoup
How can I resolve this error?