Android login/registration with shared preferences
Solution 1
This is working code, try it.
MainActivity.java
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
public class MainActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = new MenuInflater(this);
inflater.inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
switch (id) {
case R.id.menu1:
Intent intent1 = new Intent(this, Login.class);
this.startActivity(intent1);
break;
case R.id.menu2:
Intent intent2 = new Intent(this, MainActivity.class);
this.startActivity(intent2);
break;
default:
return super.onOptionsItemSelected(item);
}
return true;
}
}
Reg.java
import android.content.Intent;
import android.content.SharedPreferences;
import android.content.SharedPreferences.Editor;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class Reg extends ActionBarActivity {
SharedPreferences sharedPreferences;
Editor editor;
Button buttonReg2;
EditText txtUsername, txtPassword, txtEmail;
UserSession session;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.reg);
txtUsername = (EditText) findViewById(R.id.Name);
txtPassword = (EditText) findViewById(R.id.txtPassword);
txtEmail = (EditText) findViewById(R.id.Email);
buttonReg2 = (Button) findViewById(R.id.buttonReg2);
// creating an shared Preference file for the information to be stored
// first argument is the name of file and second is the mode, 0 is private mode
sharedPreferences = getApplicationContext().getSharedPreferences("Reg", 0);
// get editor to edit in file
editor = sharedPreferences.edit();
buttonReg2.setOnClickListener(new View.OnClickListener() {
public void onClick (View v) {
String name = txtUsername.getText().toString();
String email = txtEmail.getText().toString();
String pass = txtPassword.getText().toString();
if(txtUsername.getText().length()<=0){
Toast.makeText(Reg.this, "Enter name", Toast.LENGTH_SHORT).show();
}
else if( txtEmail.getText().length()<=0){
Toast.makeText(Reg.this, "Enter email", Toast.LENGTH_SHORT).show();
}
else if( txtPassword.getText().length()<=0){
Toast.makeText(Reg.this, "Enter password", Toast.LENGTH_SHORT).show();
}
else{
// as now we have information in string. Lets stored them with the help of editor
editor.putString("Name", name);
editor.putString("Email",email);
editor.putString("txtPassword",pass);
editor.commit();} // commit the values
// after saving the value open next activity
Intent ob = new Intent(Reg.this, Login.class);
startActivity(ob);
}
});
}
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = new MenuInflater(this);
inflater.inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
switch (id) {
case R.id.menu1:
Intent intent1 = new Intent(this, Login.class);
this.startActivity(intent1);
break;
case R.id.menu2:
Intent intent2 = new Intent(this, MainActivity.class);
this.startActivity(intent2);
break;
default:
return super.onOptionsItemSelected(item);
}
return true;
}
}
Login.java
import android.content.Context;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class Login extends ActionBarActivity {
//public static android.content.SharedPreferences SharedPreferences = null;
private static final String PREFER_NAME = "Reg";
Button buttonLogin;
EditText txtUsername, txtPassword;
// User Session Manager Class
UserSession session;
private SharedPreferences sharedPreferences;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.login);
Button switchButton = (Button)findViewById(R.id.buttonReg);
switchButton.setOnClickListener (new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent=new Intent(Login.this, Reg.class);
startActivity(intent);
}
});
// User Session Manager
session = new UserSession(getApplicationContext());
// get Email, Password input text
txtUsername = (EditText) findViewById(R.id.txtUsername);
txtPassword = (EditText) findViewById(R.id.txtPassword);
Toast.makeText(getApplicationContext(),
"User Login Status: " + session.isUserLoggedIn(),
Toast.LENGTH_LONG).show();
// User Login button
buttonLogin = (Button) findViewById(R.id.buttonLogin);
sharedPreferences = getSharedPreferences(PREFER_NAME, Context.MODE_PRIVATE);
// Login button click event
buttonLogin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
// Get username, password from EditText
String username = txtUsername.getText().toString();
String password = txtPassword.getText().toString();
// Validate if username, password is filled
if(username.trim().length() > 0 && password.trim().length() > 0){
String uName = null;
String uPassword =null;
if (sharedPreferences.contains("Name"))
{
uName = sharedPreferences.getString("Name", "");
}
if (sharedPreferences.contains("txtPassword"))
{
uPassword = sharedPreferences.getString("txtPassword", "");
}
// Object uName = null;
// Object uEmail = null;
if(username.equals(uName) && password.equals(uPassword)){
session.createUserLoginSession(uName,
uPassword);
// Starting MainActivity
Intent i = new Intent(getApplicationContext(),MainActivity.class);
i.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
// Add new Flag to start new Activity
i.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(i);
finish();
}else{
// username / password doesn't match&
Toast.makeText(getApplicationContext(),
"Username/Password is incorrect",
Toast.LENGTH_LONG).show();
}
}else{
// user didn't entered username or password
Toast.makeText(getApplicationContext(),
"Please enter username and password",
Toast.LENGTH_LONG).show();
}
}
});
}
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = new MenuInflater(this);
inflater.inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
switch (id) {
case R.id.menu1:
Intent intent1 = new Intent(this, Login.class);
this.startActivity(intent1);
break;
case R.id.menu2:
Intent intent2 = new Intent(this, MainActivity.class);
this.startActivity(intent2);
break;
default:
return super.onOptionsItemSelected(item);
}
return true;
}
}
UserSession.java
package com.example.tripmanager;
import java.util.HashMap;
import android.content.Context;
import android.content.Intent;
import android.content.SharedPreferences;
import android.content.SharedPreferences.Editor;
public class UserSession {
// Shared Preferences reference
SharedPreferences pref;
// Editor reference for Shared preferences
Editor editor;
// Context
Context _context;
// Shared preferences mode
int PRIVATE_MODE = 0;
// Shared preferences file name
public static final String PREFER_NAME = "Reg";
// All Shared Preferences Keys
public static final String IS_USER_LOGIN = "IsUserLoggedIn";
// User name (make variable public to access from outside)
public static final String KEY_NAME = "Name";
// Email address (make variable public to access from outside)
public static final String KEY_EMAIL = "Email";
// password
public static final String KEY_PASSWORD = "txtPassword";
// Constructor
public UserSession(Context context){
this._context = context;
pref = _context.getSharedPreferences(PREFER_NAME, PRIVATE_MODE);
editor = pref.edit();
}
//Create login session
public void createUserLoginSession(String uName, String uPassword){
// Storing login value as TRUE
editor.putBoolean(IS_USER_LOGIN, true);
// Storing name in preferences
editor.putString(KEY_NAME, uName);
// Storing email in preferences
editor.putString(KEY_EMAIL, uPassword);
// commit changes
editor.commit();
}
/**
* Check login method will check user login status
* If false it will redirect user to login page
* Else do anything
* */
public boolean checkLogin(){
// Check login status
if(!this.isUserLoggedIn()){
// user is not logged in redirect him to Login Activity
Intent i = new Intent(_context, Login.class);
// Closing all the Activities from stack
i.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
// Add new Flag to start new Activity
i.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
// Staring Login Activity
_context.startActivity(i);
return true;
}
return false;
}
/**
* Get stored session data
* */
public HashMap<String, String> getUserDetails(){
//Use hashmap to store user credentials
HashMap<String, String> user = new HashMap<String, String>();
// user name
user.put(KEY_NAME, pref.getString(KEY_NAME, null));
// user email id
user.put(KEY_EMAIL, pref.getString(KEY_EMAIL, null));
// return user
return user;
}
/**
* Clear session details
* */
public void logoutUser(){
// Clearing all user data from Shared Preferences
editor.clear();
editor.commit();
// After logout redirect user to MainActivity
Intent i = new Intent(_context, MainActivity.class);
// Closing all the Activities
i.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
// Add new Flag to start new Activity
i.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
// Staring Login Activity
_context.startActivity(i);
}
// Check for login
public boolean isUserLoggedIn(){
return pref.getBoolean(IS_USER_LOGIN, false);
}
}
Solution 2
This code working in my project,must try it
Use this code after login validation to store login value
String Username = textInputEditTextEmail.getText().toString();
String Password = textInputEditTextPassword.getText().toString();
final SharedPreferences sharedPref = PreferenceManager.getDefaultSharedPreferences(this);
SharedPreferences.Editor editor = sharedPref.edit();
editor.putBoolean("Registered", true);
editor.putString("Username", Username);
editor.putString("Password", Password);
editor.apply();
Create new empty activity and copy this code
Boolean Registered;
final SharedPreferences sharedPref = PreferenceManager.getDefaultSharedPreferences(this);
Registered = sharedPref.getBoolean("Registered", false);
if (!Registered)
{
startActivity(new Intent(this,Splash.class));
}else {
startActivity(new Intent(this,MainActivity.class));
}
In Menifest file copy the intent-filter code in newly created empty file for initial access.
For Logout, Create any button copy this code in click on listener
SharedPreferences preferences = PreferenceManager.getDefaultSharedPreferences(MainActivity.this);
SharedPreferences.Editor editor = preferences.edit();
editor.clear();
editor.commit();
Intent i = new Intent(MainActivity.this,Login.class);
startActivity(i);
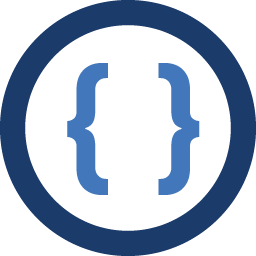
Admin
Updated on August 03, 2020Comments
-
Admin almost 4 years
I'm new to Android. I'm doing an app for an university exam. I have to do an application for a travel agency. I'd like to manage the user session with shared preferences in order to save basic information and the eventually travels that the user booked. I can't find an example on the web that combine the registration and login forms. I wrote this code but it's not working. It doesn't show any error. I think what I wrote it's not logic. I'd like to register first and login with the information I gave in the registration form. Thank you for your help.
This is my Login.java:
package com.example.trip; import android.content.Context; import android.content.Intent; import android.os.Bundle; import android.support.v7.app.ActionBarActivity; import android.view.Menu; import android.view.MenuInflater; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; public class Login extends ActionBarActivity { public static android.content.SharedPreferences SharedPreferences = null; private static final String PREFER_NAME = null; Button buttonLogin; EditText txtUsername, txtPassword; // User Session Manager Class UserSession session; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.login); Button switchButton = (Button)findViewById(R.id.buttonReg); switchButton.setOnClickListener (new View.OnClickListener() { @Override public void onClick(View v) { Intent intent=new Intent(Login.this, Reg.class); startActivity(intent); } }); // User Session Manager session = new UserSession(getApplicationContext()); // get Email, Password input text txtUsername = (EditText) findViewById(R.id.txtUsername); txtPassword = (EditText) findViewById(R.id.txtPassword); Toast.makeText(getApplicationContext(), "User Login Status: " + session.isUserLoggedIn(), Toast.LENGTH_LONG).show(); // User Login button buttonLogin = (Button) findViewById(R.id.buttonLogin); SharedPreferences = getSharedPreferences(PREFER_NAME, Context.MODE_PRIVATE); // Login button click event buttonLogin.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { // Get username, password from EditText String username = txtUsername.getText().toString(); String password = txtPassword.getText().toString(); // Validate if username, password is filled if(username.trim().length() > 0 && password.trim().length() > 0){ if (SharedPreferences.contains("name")) { String uName = (SharedPreferences).getString("name", ""); } if (SharedPreferences.contains("email")) { String uEmail = (SharedPreferences).getString("email", ""); } Object uName = null; Object uEmail = null; if(username.equals(uName) && password.equals(uEmail)){ session.createUserLoginSession(uName, uEmail); // Starting MainActivity Intent i = new Intent(getApplicationContext(),MainActivity.class); i.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP); // Add new Flag to start new Activity i.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK); startActivity(i); finish(); }else{ // username / password doesn't match& Toast.makeText(getApplicationContext(), "Username/Password is incorrect", Toast.LENGTH_LONG).show(); } }else{ // user didn't entered username or password Toast.makeText(getApplicationContext(), "Please enter username and password", Toast.LENGTH_LONG).show(); } } }); } public boolean onCreateOptionsMenu(Menu menu) { MenuInflater inflater = new MenuInflater(this); inflater.inflate(R.menu.main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { int id = item.getItemId(); switch (id) { case R.id.MENU_1: Intent intent1 = new Intent(this, Login.class); this.startActivity(intent1); break; case R.id.MENU_2: Intent intent2 = new Intent(this, MainActivity.class); this.startActivity(intent2); break; default: return super.onOptionsItemSelected(item); } return true; } }
This is the UserSession.java:
package com.example.trip; import java.util.HashMap; import android.content.Context; import android.content.Intent; import android.content.SharedPreferences; import android.content.SharedPreferences.Editor; public class UserSession { // Shared Preferences reference SharedPreferences pref; // Editor reference for Shared preferences Editor editor; // Context Context _context; // Shared preferences mode int PRIVATE_MODE = 0; // Shared preferences file name public static final String PREFER_NAME = "AndroidExamplePref"; // All Shared Preferences Keys public static final String IS_USER_LOGIN = "IsUserLoggedIn"; // User name (make variable public to access from outside) public static final String KEY_NAME = "name"; // Email address (make variable public to access from outside) public static final String KEY_EMAIL = "email"; // Constructor public UserSession(Context context){ this._context = context; pref = _context.getSharedPreferences(PREFER_NAME, PRIVATE_MODE); editor = pref.edit(); } //Create login session public void createUserLoginSession(Object uName, Object uEmail){ // Storing login value as TRUE editor.putBoolean(IS_USER_LOGIN, true); // Storing name in preferences editor.putString(KEY_NAME, (String) uName); // Storing email in preferences editor.putString(KEY_EMAIL, (String) uEmail); // commit changes editor.commit(); } /** * Check login method will check user login status * If false it will redirect user to login page * Else do anything * */ public boolean checkLogin(){ // Check login status if(!this.isUserLoggedIn()){ // user is not logged in redirect him to Login Activity Intent i = new Intent(_context, Login.class); // Closing all the Activities from stack i.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP); // Add new Flag to start new Activity i.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK); // Staring Login Activity _context.startActivity(i); return true; } return false; } /** * Get stored session data * */ public HashMap<String, String> getUserDetails(){ //Use hashmap to store user credentials HashMap<String, String> user = new HashMap<String, String>(); // user name user.put(KEY_NAME, pref.getString(KEY_NAME, null)); // user email id user.put(KEY_EMAIL, pref.getString(KEY_EMAIL, null)); // return user return user; } /** * Clear session details * */ public void logoutUser(){ // Clearing all user data from Shared Preferences editor.clear(); editor.commit(); // After logout redirect user to MainActivity Intent i = new Intent(_context, MainActivity.class); // Closing all the Activities i.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP); // Add new Flag to start new Activity i.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK); // Staring Login Activity _context.startActivity(i); } // Check for login public boolean isUserLoggedIn(){ return pref.getBoolean(IS_USER_LOGIN, false); } }
This is the MainActivity.java:
public class MainActivity extends ActionBarActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public boolean onCreateOptionsMenu(Menu menu) { MenuInflater inflater = new MenuInflater(this); inflater.inflate(R.menu.main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { int id = item.getItemId(); switch (id) { case R.id.MENU_1: Intent intent1 = new Intent(this, Login.class); this.startActivity(intent1); break; case R.id.MENU_2: Intent intent2 = new Intent(this, MainActivity.class); this.startActivity(intent2); break; default: return super.onOptionsItemSelected(item); } return true; } }
This is my Reg.java:
package com.example.trip; import android.content.Intent; import android.content.SharedPreferences; import android.content.SharedPreferences.Editor; import android.os.Bundle; import android.support.v7.app.ActionBarActivity; import android.view.Menu; import android.view.MenuInflater; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; import android.view.View.OnClickListener; public class Reg extends ActionBarActivity { SharedPreferences SharedPreferences; Editor editor; Button buttonReg2; EditText txtUsername, txtPassword, txtEmail; UserSession session; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.reg); txtUsername = (EditText) findViewById(R.id.Name); txtPassword = (EditText) findViewById(R.id.txtPassword); txtEmail = (EditText) findViewById(R.id.Email); buttonReg2 = (Button) findViewById(R.id.buttonReg2); // creating an shared Preference file for the information to be stored // first argument is the name of file and second is the mode, 0 is private mode SharedPreferences = getSharedPreferences("Reg", 0); // get editor to edit in file editor = SharedPreferences.edit(); buttonReg2.setOnClickListener(new View.OnClickListener() { public void onClick (View v) { String name = txtUsername.getText().toString(); String email = txtEmail.getText().toString(); String pass = txtPassword.getText().toString(); if(txtUsername.getText().length()<=0){ Toast.makeText(Reg.this, "Enter name", Toast.LENGTH_SHORT).show(); } else if( txtEmail.getText().length()<=0){ Toast.makeText(Reg.this, "Enter email", Toast.LENGTH_SHORT).show(); } else if( txtPassword.getText().length()<=0){ Toast.makeText(Reg.this, "Enter password", Toast.LENGTH_SHORT).show(); } else{ // as now we have information in string. Lets stored them with the help of editor editor.putString("Name", name); editor.putString("Email",email); editor.putString("txtPassword",pass); editor.commit();} // commit the values // after saving the value open next activity Intent ob = new Intent(Reg.this, Login.class); startActivity(ob); } }); } public boolean onCreateOptionsMenu(Menu menu) { MenuInflater inflater = new MenuInflater(this); inflater.inflate(R.menu.main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { int id = item.getItemId(); switch (id) { case R.id.MENU_1: Intent intent1 = new Intent(this, Login.class); this.startActivity(intent1); break; case R.id.MENU_2: Intent intent2 = new Intent(this, MainActivity.class); this.startActivity(intent2); break; default: return super.onOptionsItemSelected(item); } return true; } }
The XML code of the MainActivity:
<?xml version="1.0" encoding="utf-8"?> <TableLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="top" android:shrinkColumns="0" android:stretchColumns="1" > <TableRow android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="fill_horizontal|center_vertical" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="fill_horizontal|center_vertical" android:text="@string/destinazione" /> <EditText android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="text" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="fill_horizontal|center_vertical" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="fill_horizontal|center_vertical" android:text="@string/checkin" /> <EditText android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="date" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="fill_horizontal|center_vertical" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="fill_horizontal|center_vertical" android:text="@string/checkout" /> <EditText android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="date" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="fill_horizontal|center_vertical" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="fill_horizontal|center_vertical" android:text="@string/viaggiatori" /> <EditText android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="number" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="fill_horizontal|center_vertical" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="fill_horizontal|center_vertical" android:text="@string/stelle" /> <Spinner android:id="@+id/spinner" android:layout_width="match_parent" android:layout_height="match_parent" android:entries="@array/stelle"/> </TableRow> <Button android:id="@+id/buttonFind" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/buttonFind" /> </TableLayout>
The XML code for the login form:
<?xml version="1.0" encoding="utf-8"?> <TableLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="top" android:shrinkColumns="0" android:stretchColumns="1" > <TableRow android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="fill_horizontal|center_vertical" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="fill_horizontal|center_vertical" android:text="@string/txtUsername" /> <EditText android:id="@+id/txtUsername" android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="text" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="fill_horizontal|center_vertical" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="fill_horizontal|center_vertical" android:text="@string/txtPassword" /> <EditText android:id="@+id/txtPassword" android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="textPassword" /> </TableRow> <Button android:id="@+id/buttonLogin" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/buttonLogin" /> <Button android:id="@+id/buttonReg" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/buttonReg" /> </TableLayout>
This is the XML code for the registration form:
<?xml version="1.0" encoding="utf-8"?> <TableLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="top" android:shrinkColumns="0" android:stretchColumns="1" > <TableRow android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="fill_horizontal|center_vertical" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="fill_horizontal|center_vertical" android:text="@string/Name" /> <EditText android:id="@+id/Name" android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="text" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="fill_horizontal|center_vertical" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="fill_horizontal|center_vertical" android:text="@string/Email" /> <EditText android:id="@+id/Email" android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="textEmailAddress" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="fill_horizontal|center_vertical" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="fill_horizontal|center_vertical" android:text="@string/txtPassword" /> <EditText android:id="@+id/txtPassword" android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="textPassword" /> </TableRow> <Button android:id="@+id/buttonReg2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/buttonReg2" /> </TableLayout>
-
Admin over 9 yearsI edit the question I did. I made the changes you write me but it still doesn't working.
-
Blue_Alien over 9 yearsLogin.jadva, Line 19 : private static final String PREFER_NAME = "AndroidExamplePref";
-
Admin over 9 yearsStill not working. When I touch the button 'Register me' nothing happen!
-
Admin over 9 yearsI put "public static final" instead of private and after the registration it opens the login form, but the login doesn't recognize username and password.
-
Blue_Alien over 9 yearsedited the answer: be careful about using object name! its start with lower case letter 's', not 'S'.
-
Admin over 9 yearsyes, i named it Reg, but i don't know where i have to use that name in usersession.java and login.java
-
Blue_Alien over 9 yearsthe variable names are also different: using "Name" to gegister sharedpreference, but using "name" to retrieve. and the same for email. do u wnt to compare the three parameters forlogin?
-
Admin over 9 yearsI'd like to register with name, email and password and then login with only name and password.
-
Blue_Alien over 9 yearsworking code added for your reference, try it, its tested, if you get any error plese put it over here.
-
Admin over 9 yearsThank you! It's working. Where can I see the saved data? And how can I manage unique session? I don't want two users with the same name and email..