android - MediaRecorder throws illegalstateexception
Solution 1
The problem isn't in stop, but in start. It doesn't start correctly, that's why you can't stop it later.
Check couple of things: a) That you added persmission android.permission.RECORD_AUDIO
b) That you write to SD card (I am not sure it's requirement, but I believe I had problem writing to internal memory). You will need permission to write to SD card.
c) Also, try to set onErrorListener http://developer.android.com/reference/android/media/MediaPlayer.html#setOnErrorListener(android.media.MediaPlayer.OnErrorListener)
d) Try to reset() MediaPlayer before any other calls I would recommend to read this article http://developer.android.com/reference/android/media/MediaPlayer.html#Valid_and_Invalid_States Android's MediaRecorder states is the nightmare.
Solution 2
It looks like that error is thrown when you call stop() from an invalid state.
The activity cycle for the recorder also seems to indicate you need to prepare() it before you can start recording - are you doing that? stop() isn't a valid command unless the recorder is at least prepared.
If you are, maybe it takes a moment for the recorder to actually settle in a valid state before you can later call stop(). You are calling it immediately after start() so maybe something is happening there.
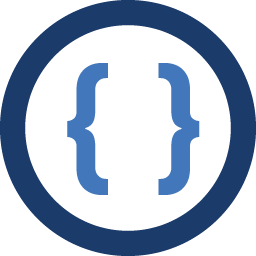
Admin
Updated on June 23, 2022Comments
-
Admin about 2 years
I am trying to develop a simple android audio recorder.Everything builds fine and it also runs fine on the android device. It seems like i can start the recording but when i want to stop it throws a IllegalStateException. I can't find the mistake. Here is the code:
public class VoiceRecorder { MediaRecorder recorder= new MediaRecorder(); static Context cont; public void startRecord(Context context) throws IllegalStateException, IOException{ cont = context; recorder.setAudioSource(MediaRecorder.AudioSource.MIC); recorder.setOutputFormat(MediaRecorder.OutputFormat.THREE_GPP); recorder.setAudioEncoder(MediaRecorder.AudioEncoder.AMR_NB); recorder.setOutputFile(cont.getFilesDir()+"/recordings.3gp"); recorder.prepare(); recorder.start(); } public void stopRecording(Context context) { cont = context; recorder.stop(); recorder.release(); File file = new File (cont.getFilesDir()+"/recordings.3gp"); UploadFile.uploadFile("recordings.3gp", file); recorder = null; } }
I want to trigger it with:
VoiceRecorder vr = new VoiceRecorder();
vr.startRecord(cont);
vr.stopRecording(cont);
when calling start Logcat says: (what should be ok)
09-06 22:56:42.830: D/AudioHardwareMSM72XX(123): audpre_index = 0, tx_iir_index = 0 09-06 22:56:42.840: D/HTC Acoustic(123): msm72xx_enable_audpre: 0x0000 09-06 22:56:42.850: I/AudioHardwareMSM72XX(123): Routing audio to Speakerphone 09-06 22:56:42.850: D/HTC Acoustic(123): msm72xx_enable_audpp: 0x0001 09-06 22:56:42.850: I/AudioHardwareMSM72XX(123): Routing audio to Speakerphone 09-06 22:56:42.860: D/HTC Acoustic(123): msm72xx_enable_audpp: 0x0001 09-06 22:56:42.870: D/AudioFlinger(123): setParameters(): io 3, keyvalue routing=262144;vr_mode=0, tid 156, calling tid 123 09-06 22:56:42.870: I/AudioHardwareMSM72XX(123): Routing audio to Speakerphone 09-06 22:56:42.880: D/AudioHardwareMSM72XX(123): audpre_index = 0, tx_iir_index = 0 09-06 22:56:42.880: D/HTC Acoustic(123): msm72xx_enable_audpre: 0x0000 09-06 22:56:42.880: I/AudioHardwareMSM72XX(123): do input routing device 40000 09-06 22:56:42.880: I/AudioHardwareMSM72XX(123): Routing audio to Speakerphone 09-06 22:56:42.890: D/HTC Acoustic(123): msm72xx_enable_audpp: 0x0001
But when i call stop:
09-06 22:59:52.440: E/MediaRecorder(1069): stop called in an invalid state: 1 09-06 22:59:52.440: W/System.err(1069): java.lang.IllegalStateException 09-06 22:59:52.460: W/System.err(1069): at android.media.MediaRecorder.stop(Native Method) 09-06 22:59:52.460: W/System.err(1069): at de.spyapp.VoiceRecorder.stopRecording(VoiceRecorder.java:33) 09-06 22:59:52.460: W/System.err(1069): at de.spyapp.CheckCMD.checkCMD(CheckCMD.java:30) 09-06 22:59:52.460: W/System.err(1069): at de.spyapp.AppActivity$2.run(AppActivity.java:44) 09-06 22:59:52.460: W/System.err(1069): at java.lang.Thread.run(Thread.java:1096)
-
TheMaster42 almost 12 yearsNot allowed to post more than two hyperlinks. Some further reading: - stackoverflow.com/questions/11852852/… - benmccann.com/dev-blog/android-audio-recording-tutorial
-
Admin almost 12 yearsall permissions are ok. i tried changing to sd card and did a reset at beginning of startrecording method. Now logcat tells me sth new on .start(): 09-06 23:34:20.310: D/audio_input(123): DoStop: X 09-06 23:34:20.330: D/audio_input(123): DoReset: E 09-06 23:34:20.340: D/audio_input(123): DoReset: X 09-06 23:34:20.340: E/audio_input(123): unsupported parameter: x-pvmf/media-input-node/cap-config-interface;valtype=key_specific_value 09-06 23:34:20.340: E/audio_input(123): VerifyAndSetParameter failed
-
Admin almost 12 yearshmm i called the prepare before start and i'm waiting like 20sec untill i call the stop
-
Neil C. Obremski over 8 yearsThis answer is referencing
MediaPlayer
but the OP is asking aboutMediaRecorder