android : NestedScrollview bottom two button attached
Solution 1
You can use Relativelayout
as your outer layout and keep CoordinatorLayout
inside it, make Two buttons android:layout_alignParentBottom="true"
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<android.support.design.widget.CoordinatorLayout
android:id="@+id/coordinate"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_above="@+id/groupbutton"
android:fitsSystemWindows="true"
tools:context="com.chivazo.chivazoandroid.activities.SingleProductActivity">
<android.support.design.widget.AppBarLayout
android:id="@+id/app_bar"
android:layout_width="match_parent"
android:layout_height="@dimen/app_bar_height"
android:fitsSystemWindows="true"
android:theme="@style/AppTheme.AppBarOverlay">
<android.support.design.widget.CollapsingToolbarLayout
android:id="@+id/toolbar_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
app:contentScrim="?attr/colorPrimary"
app:expandedTitleMarginBottom="30dp"
app:expandedTitleMarginEnd="64dp"
app:expandedTitleMarginStart="48dp"
app:layout_scrollFlags="scroll|exitUntilCollapsed">
<ImageView
android:id="@+id/backdrop"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
android:scaleType="centerCrop"
android:src="@drawable/a"
app:layout_collapseMode="parallax" />
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
app:layout_collapseMode="pin"
app:popupTheme="@style/AppTheme.PopupOverlay" />
</android.support.design.widget.CollapsingToolbarLayout>
</android.support.design.widget.AppBarLayout>
<include layout="@layout/content_single_product" />
</android.support.design.widget.CoordinatorLayout>
<LinearLayout
android:id="@+id/groupbutton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_gravity="bottom"
android:orientation="horizontal"
android:weightSum="2">
<android.support.v7.widget.AppCompatButton
android:id="@+id/share"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="share" />
<android.support.v7.widget.AppCompatButton
android:id="@+id/go_wishlist"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="go to Wishlist" />
</LinearLayout>
</RelativeLayout>
Solution 2
The app:layout_anchor="@id/anchor_view"
and
app:layout_anchorGravity="bottom"
are the Important attributes here
<android.support.design.widget.CoordinatorLayout
android:id="@+id/coordinator_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
tools:context=".ProductDetailActivity">
<android.support.design.widget.AppBarLayout
android:id="@+id/app_bar"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.design.widget.CollapsingToolbarLayout
android:id="@+id/collapsing_toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_scrollFlags="scroll">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="@color/colorPrimary"/>
</android.support.design.widget.CollapsingToolbarLayout>
</android.support.design.widget.AppBarLayout>
<android.support.v4.widget.NestedScrollView
android:id="@+id/nested_scroll"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
.
.
.
<View
android:id="@+id/anchor_view"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
.
.
.
.
</android.support.v4.widget.NestedScrollView>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
app:layout_anchor="@id/anchor_view"
app:layout_anchorGravity="bottom">
<Button
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"/>
<Button
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"/>
</LinearLayout>
</android.support.design.widget.CoordinatorLayout>
Solution 3
Simply add your layout in Coordinatorlayout below NestedScrollView in xml and do the following in java.
CoordinatorLayout.LayoutParams layoutParams = (CoordinatorLayout.LayoutParams) layoutToBeAnchored.getLayoutParams();
layoutParams.setAnchorId(R.id.layoutBelowWhichToBeAnchored);
layoutParams.anchorGravity = Gravity.CENTER;
layoutToBeAnchored.setLayoutParams(layoutParams);
Hope this helps you guys. For any reference you can check the example at - https://github.com/IsUncommon/Droidcon-India-2015
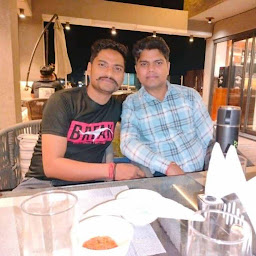
Sonu Kumar
I came across your profile Project and feel with your background I would be a great fit for an exciting Android App Developer position. I would love to chat with you about this opportunity a bit more. I explain the details below. More Experience in Android Apps Application Real-Time Android Apps Create UI and Tested Performance and Optimization Apps Design Clean Architecture Components (RxJava, Room Database, Kotlin, Java, Retrofit, Dagger) Design MVP, MVVM pattern Localization Apps Module Base Instant App Bundle-Based App Sonu Kumar +919810659036 | [email protected]
Updated on June 15, 2022Comments
-
Sonu Kumar almost 2 years
I am trying to create Android product layout. In footer have two buttons it is fixed button. you can see images when I am scrolling up bottom two buttons attached in my layout. Please help how can i do?
Image 1
Image 2
after scrolling
xml
<?xml version="1.0" encoding="utf-8"?> <android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/coordinate" android:layout_width="match_parent" android:layout_height="match_parent" android:fitsSystemWindows="true" tools:context="com.chivazo.chivazoandroid.activities.SingleProductActivity"> <android.support.design.widget.AppBarLayout android:id="@+id/app_bar" android:layout_width="match_parent" android:layout_height="@dimen/app_bar_height" android:fitsSystemWindows="true" android:theme="@style/AppTheme.AppBarOverlay"> <android.support.design.widget.CollapsingToolbarLayout android:id="@+id/toolbar_layout" android:layout_width="match_parent" android:layout_height="match_parent" android:fitsSystemWindows="true" app:contentScrim="?attr/colorPrimary" app:expandedTitleMarginBottom="30dp" app:expandedTitleMarginEnd="64dp" app:expandedTitleMarginStart="48dp" app:layout_scrollFlags="scroll|exitUntilCollapsed"> <ImageView android:id="@+id/backdrop" android:layout_width="match_parent" android:layout_height="match_parent" android:fitsSystemWindows="true" android:scaleType="centerCrop" android:src="@drawable/a" app:layout_collapseMode="parallax" /> <android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" app:layout_collapseMode="pin" app:popupTheme="@style/AppTheme.PopupOverlay" /> </android.support.design.widget.CollapsingToolbarLayout> </android.support.design.widget.AppBarLayout> <include layout="@layout/content_single_product" /> <LinearLayout android:id="@+id/groupbutton" android:weightSum="2" android:orientation="horizontal" android:layout_gravity="bottom" android:layout_width="match_parent" android:layout_height="wrap_content" > <android.support.v7.widget.AppCompatButton android:layout_weight="1" android:id="@+id/share" android:text="share" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <android.support.v7.widget.AppCompatButton android:layout_weight="1" android:id="@+id/go_wishlist" android:text="go to Wishlist" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout> </android.support.design.widget.CoordinatorLayout>
-
rakesh kashyap over 7 years@SonuKumar can you please help to post the way in which you were able to achieve this
-
Sonu Kumar over 7 yearsstackoverflow.com/questions/32465548/… check this link appbarlayout app:layout_behaviour
-
Sonu Kumar over 7 yearsthanks coordinator layout already has two view not required other view
-
Admin over 7 years@SonuKumar, but I guess this solves your purpose right ?
-
Rajesh Ujade over 7 years@AnkitPachouri Thanks
-
iBug about 6 yearsThank you for this code snippet, which might provide some limited, immediate help. A proper explanation would greatly improve its long-term value by showing why this is a good solution to the problem, and would make it more useful to future readers with other, similar questions. Please edit your answer to add some explanation, including the assumptions you've made.