Android: Refresh ArrayAdapter on resume
Solution 1
//made changes in your oncreate method, see below
DBConfig info;
@Override
protected void onCreate(Bundle savedInstanceState) {
// TODO Auto-generated method stub
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
// Start db view of whiskey
info = new DBConfig(this);
whiskeylist = (ListView) findViewById(R.id.listofWhiskey);
whiskeylist.setOnItemClickListener(this);
}// end onCreate
another method give below, call this method from on ActivityResult(), but before doing that ensure that the field you added with another activity also saved into database. call show Data() from onActivityResult method or from onResume()
private void showData()
{
info.open();
DataArryWhiskey = info.getDataInArray();
info.close();
whiskeylist.setAdapter(new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, DataArryWhiskey));
}
Solution 2
Get a class level variable of ArrayAdapter adapter;
and initialize it like this way:
adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, DataArryWhiskey);
hiskeylist.setAdapter(adapter);
and inside onResume()
do like this way.
public void onResume()
{
super.onResume();
info.open();
DataArryWhiskey = info.getDataInArray();
info.close();
adapter.notifyDataSetChanged(); // refresh adapter
}
My Recommendation:
Since you are getting your values from database, use SimpleCursorAdapter
instead of ArrayAdapter
. which will do lots of other work also for you.
Here is a tutorial how to use SimpleCursorAdapter
Solution 3
You can try using adapter.clear();
before adding items.
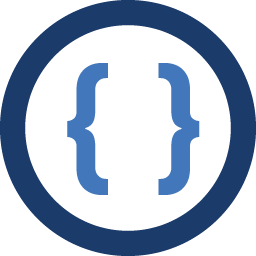
Admin
Updated on June 04, 2022Comments
-
Admin about 2 years
I am trying to create a refesh function for my main page. I have searched many site but i can't seem to find a (for me) acccesseble exaple. I am loading information from a sQLLite database. When i use my add activity and i return to the MainScreen activity the item i have added do not appear. How could i refresh this data the moment de activity is resumed.
Any help is welcome, thx in advance.
public ListView whiskeylist; String[] DataArryWhiskey; @Override protected void onCreate(Bundle savedInstanceState) { // TODO Auto-generated method stub super.onCreate(savedInstanceState); setContentView(R.layout.main); // Start db view of whiskey DBConfig info = new DBConfig(this); info.open(); DataArryWhiskey = info.getDataInArray(); info.close(); whiskeylist = (ListView) findViewById(R.id.listofWhiskey); whiskeylist.setOnItemClickListener(this); whiskeylist.setAdapter(new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, DataArryWhiskey)); }// end onCreate
On The advice of Adil i change the code to
public ListView whiskeylist; String[] DataArryWhiskey; ListAdapter Adapter; @Override protected void onCreate(Bundle savedInstanceState) { // TODO Auto-generated method stub super.onCreate(savedInstanceState); setContentView(R.layout.main); // Start db view of whiskey DBConfig info = new DBConfig(this); info.open(); DataArryWhiskey = info.getDataInArray(); info.close(); whiskeylist = (ListView) findViewById(R.id.listofWhiskey); Adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, DataArryWhiskey); whiskeylist.setAdapter(Adapter); // End db view of whiskey }// end onCreate @Override public void onResume() { super.onResume(); DBConfig info = new DBConfig(this); info.open(); DataArryWhiskey = info.getDataInArray(); info.close(); Adapter.notifyDataSetChanged(); // refresh adapter }
however i get a error on notifyDataSetChanged "the method notifyDataSetChanged is undefined for the type ListAdapter" <- fixed it by changing the ListAdapter to ArrayAdapter but the app still crashes.