Android TextView Justify Text
Solution 1
I do not believe Android supports full justification.
UPDATE 2018-01-01: Android 8.0+ supports justification modes with TextView
.
Solution 2
The @CommonsWare answer is correct. Android 8.0+ does support "Full Justification" (or simply "Justification", as it is sometimes ambiguously referred to).
Android also supports "Flush Left/Right Text Alignment". See the wikipedia article on Justification for the distinction. Many people consider the concept of 'justification' to encompass full-justification as well as left/right text alignment, which is what they end up searching for when they want to do left/right text alignment. This answer explains how to achieve the left/right text alignment.
It is possible to achieve Flush Left/Right Text Alignment (as opposed to Full Justification, as the question is asking about). To demonstrate I will be using a basic 2-column form (labels in the left column and text fields in the right column) as an example. In this example the text in the labels in the left column will be right-aligned so they appear flush up against their text fields in the right column.
In the XML layout you can get the TextView elements themselves (the left column) to align to the right by adding the following attribute inside all of the TextViews:
<TextView
...
android:layout_gravity="center_vertical|end">
...
</TextView>
However, if the text wraps to multiple lines, the text would still be flush left aligned inside the TextView. Adding the following attribute makes the actual text flush right aligned (ragged left) inside the TextView:
<TextView
...
android:gravity="end">
...
</TextView>
So the gravity attribute specifies how to align the text inside the TextView layout_gravity specifies how to align/layout the TextView element itself.
Solution 3
TextView
in Android O offers full justification (new typographic alignment) itself.
You just need to do this:
Kotlin
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
textView.justificationMode = JUSTIFICATION_MODE_INTER_WORD
}
Java
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
textView.setJustificationMode(JUSTIFICATION_MODE_INTER_WORD);
}
XML
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:justificationMode="inter_word" />
Default is JUSTIFICATION_MODE_NONE
(none
in xml).
Solution 4
To justify text in android I used WebView
setContentView(R.layout.main);
WebView view = new WebView(this);
view.setVerticalScrollBarEnabled(false);
((LinearLayout)findViewById(R.id.inset_web_view)).addView(view);
view.loadData(getString(R.string.hello), "text/html; charset=utf-8", "utf-8");
and html.
<string name="hello">
<![CDATA[
<html>
<head></head>
<body style="text-align:justify;color:gray;background-color:black;">
Lorem ipsum dolor sit amet, consectetur
adipiscing elit. Nunc pellentesque, urna
nec hendrerit pellentesque, risus massa
</body>
</html>
]]>
</string>
I can't yet upload images to prove it but "it works for me".
Solution 5
UPDATED
We have created a simple class for this. There are currently two methods to achieve what you are looking for. Both require NO WEBVIEW and SUPPORTS SPANNABLES.
LIBRARY: https://github.com/bluejamesbond/TextJustify-Android
SUPPORTS: Android 2.0 to 5.X
SETUP
// Please visit Github for latest setup instructions.
SCREENSHOT
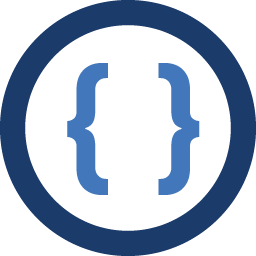
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
How do you get the text of a
TextView
to be Justified (with text flush on the left- and right- hand sides)?I found a possible solution here, but it does not work (even if you change vertical-center to center_vertical, etc).