Android Turn on/off Camera Flash Programmatically with Camera2
Solution 1
I'll be addressing you to the Android Dev. documentation about the CameraManager since more code will be required but this is the basic code to activate the Flash on the camera in API's above 21.
CameraManager camManager = (CameraManager) getSystemService(Context.CAMERA_SERVICE);
String cameraId = camManager.getCameraIdList()[0]; // Usually front camera is at 0 position.
camManager.setTorchMode(cameraId, true);
Android CameraManager documentation.
Android.hardware.camera2 documentation.
very important thing to remember that you will need to use try/catch to discard possible errors and ofcourse check that no other higher priority application is using the camera at the moment.
Solution 2
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
mCameraManager = (CameraManager) context.getSystemService(Context.CAMERA_SERVICE);
try {
for (String camID : mCameraManager.getCameraIdList()) {
CameraCharacteristics cameraCharacteristics = mCameraManager.getCameraCharacteristics(camID);
int lensFacing = cameraCharacteristics.get(CameraCharacteristics.LENS_FACING);
if (lensFacing == CameraCharacteristics.LENS_FACING_FRONT && cameraCharacteristics.get(CameraCharacteristics.FLASH_INFO_AVAILABLE)) {
mCameraId = camID;
break;
} else if (lensFacing == CameraCharacteristics.LENS_FACING_BACK && cameraCharacteristics.get(CameraCharacteristics.FLASH_INFO_AVAILABLE)) {
mCameraId = camID;
}
}
if (mCameraId != null) {
mCameraManager.setTorchMode(mCameraId, true);
}
} catch (CameraAccessException e) {
e.printStackTrace();
}
}
mCameraId will preferably turn on front camera flash by priority and if there is no front flash then back camera flash will be used. If device has no flash then mCameraId will be null and setTorchMode will not be called in the code above.
Solution 3
Try this. Is working fine on me for android Pie and above. There is a toggle button that turns on and off the flashlight
ToggleButton FlB = (ToggleButton) findViewById(R.id.FlashBt);
final boolean[] IsTurnedOn = {false};
final CameraManager camManager = (CameraManager) getSystemService(Context.CAMERA_SERVICE);
String cameraId = null; // Usually front camera is at 0 position.
try {
cameraId = camManager.getCameraIdList()[0];
} catch (CameraAccessException e) {
e.printStackTrace();
}
final String finalCameraId = cameraId;
FlB.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!IsTurnedOn[0]){
try {
camManager.setTorchMode(finalCameraId, true);
} catch (CameraAccessException e) {
e.printStackTrace();
}
}
else {
try {
camManager.setTorchMode(finalCameraId, false);
} catch (CameraAccessException e) {
e.printStackTrace();
}
}
IsTurnedOn[0] =!IsTurnedOn[0];
}
});
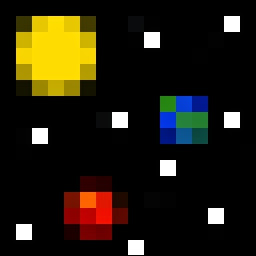
Comments
-
Benjamin Owen almost 2 years
I am making a simple application that acts as a flashlight. I need to be able to turn on and off the flash from a button in the application with the Camera2 API, as I get errors with the old camera API. I would like to use a "torch" flashlight, where the camera doesn't have to open in order for the flash to turn on. I know it's possible, as lots of phones have a flash on/off in the quick settings menu, but I can't find any code or tutorials about how to do this.
Code:
Camera cam = Camera.open(); Parameters p = cam.getParameters(); p.setFlashMode(Parameters.FLASH_MODE_TORCH); cam.setParameters(p); cam.startPreview();
I am running on a Nexus 6P with Android 6.0.1.
EDIT: I need to use the Camera2 API. I haven't found a good tutorial for this yet, and after getting an answer, I will create one in Q/A format on here.