Angular 5 disable a table row
16,343
Solution 1
What do you mean with disable? If you want to remove the list item do this on the html :
<table class="table m-table m-table--head-bg-metal">
<thead>
<tr>
<th>
Nom
</th>
<th>
Prénom
</th>
<th>
Email
</th>
<th>
Action
</th>
</tr>
</thead>
<tbody>
<tr *ngIf="!isDisable">
<td>
Jhon
</td>
<td>
Stone
</td>
<td>
[email protected]
</td>
<td>
<button class="btn btn-outline-danger m-btn m-btn--icon btn-sm m-btn--icon-only m-btn--pill m-btn--air" (click)="onDisableUser()">
<i class="fa fa-close"></i>
</button>
</td>
</tr>
</tbody>
</table>
And on the typescript:
isDisable = false;
onDisableUser(){
this.isDisable = true;
}
If you want to disable it with css use this in html:
<tr [ngClass]="{'disabled': isDisable}>
Solution 2
You can use the css method to disable the table row.
Set the disabled to true when clicked and set the class to the <tr>
if disabled=true
.disable{
cursor: not-allowed;
}
<table>
<tr class="disable">
<td> akjsd </td>
</tr>
<tr >
<td> akjsd </td>
</tr>
</table>
isDisable = false;
onDisableUser(){
this.isDisable = true;
}
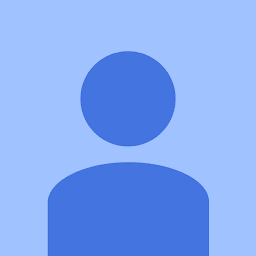
Author by
antony
Updated on December 05, 2022Comments
-
antony over 1 year
This is my HTML code for my table to display list of users:
<table class="table m-table m-table--head-bg-metal"> <thead> <tr> <th> Nom </th> <th> Prénom </th> <th> Email </th> <th> Action </th> </tr> </thead> <tbody> <tr> <td> Jhon </td> <td> Stone </td> <td> [email protected] </td> <td> <button class="btn btn-outline-danger m-btn m-btn--icon btn-sm m-btn--icon-only m-btn--pill m-btn--air" (click)="onDisableUser()"> <i class="fa fa-close"></i> </button> </td> </tr> </tbody>
This is typescript method:
onDisableUser(){ //this is the method to disable the table row.. }
What I want is when I click () on the action button, the table row will be disabled.
Thanks in advance.
-
Srigar almost 6 yearsWhether row should be disabled or button inside td? Because all td are plain text only. Explain clearly
-
antony almost 6 yearsWhen I click on the button, I want to disable the whole <tr></tr>
-
Naveen Yadav almost 6 yearsWhen you disable a button it becomes non-clickable, but I don't understand what do you mean by disabling the 'row'.
-
Niladri almost 6 years@antony you can only disable the row if there are input fields .. by default there are only labels which are read only.
-
Pardeep Jain almost 6 yearswhat do you mean by
disabled
here , are you clear with your requirements? -
antony almost 6 yearscheck this screenShot i.stack.imgur.com/ZQvO0.png
-
antony almost 6 yearsWhat I want is very simple: click on the button => disable the table row
-
Srigar almost 6 yearsSimple, on click button add css dynamically based on condition and in css simply add { pointer-events:none; opacity: 0.5}
-
antony almost 6 years@Srigar Thanks men ;) here we are (y)
-
-
antony almost 6 yearsthis code will totally disappeared the tag I want just to disabled check this screenshot : i.stack.imgur.com/ZQvO0.png
-
Tiago Machado almost 6 yearsuse the second option create a css class disabled and set opacity:0,5 and pointer-events:none