Angular 6 - No 'Access-Control-Allow-Origin' header is present on the requested resource
19,207
Solution 1
If you are using Express, you can try this cors package.
EDIT:
var express = require('express')
var cors = require('cors')
var app = express()
app.use(cors())
app.get('/products/:id', function (req, res, next) {
res.json({msg: 'This is CORS-enabled for all origins!'})
})
app.listen(80, function () {
console.log('CORS-enabled web server listening on port 80')
})
Solution 2
Great! you need to enable domain CORS that can make requests! You can try that
app.use(function(req, res, next) {
res.header("Access-Control-Allow-Origin", "*");
res.header("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
next();
});
"Access-Control-Allow-Origin", "*" -> used to accept all domains
Or you can just set localhost:4200
or something like that
Try that and tell me if worked! Thanks! Hope this helps!
Related videos on Youtube
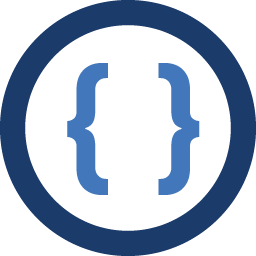
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have an Angular 6 project, which has a service with is pointing to a server.js
Angular is on port: 4200 and Server.js is on port: 3000.
When I point the service to
http://localhost:3000/api/posts
(Server.js location), I'm getting this error:Failed to load http://localhost:3000/api/posts: No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:4200' is therefore not allowed access.
Here is the server.js code:
// Get dependencies const express = require('express'); const path = require('path'); const http = require('http'); const bodyParser = require('body-parser'); // Get our API routes const api = require('./server/routes/api'); const app = express(); // Parsers for POST data app.use(bodyParser.json()); app.use(bodyParser.urlencoded({ extended: false })); // Point static path to dist app.use(express.static(path.join(__dirname, 'dist'))); // Set our api routes app.use('/api', api); // Catch all other routes and return the index file app.get('*', (req, res) => { res.sendFile(path.join(__dirname, 'dist/myproject/index.html')); }); /** * Get port from environment and store in Express. */ const port = process.env.PORT || '3000'; app.set('port', port); /** * Create HTTP server. */ const server = http.createServer(app); /** * Listen on provided port, on all network interfaces. */ server.listen(port, () => console.log(`API running on localhost:${port}`));
My question is:
How to I get server.js to allow this call?
-
AmirReza-Farahlagha over 4 yearsThis answer maybe useful: stackoverflow.com/a/58064366/7059557
-
-
Admin almost 6 yearsI added this to my server.js code and restarted it but still the same error. I don't need to add the last line right?
-
Chiien almost 6 yearsRight! Sorry, last line just for explain! But didn't working? =(
-
Chiien almost 6 yearsTry that! stackoverflow.com/questions/18310394/…
-
Javascript Hupp Technologies almost 6 years@PaulB You added this code at first after
const app = express();
? -
Admin almost 6 yearsStill same error and when I change server.js to port 4200 (same as angular) I get error: Uncaught SyntaxError: Unexpected token <
-
mittal bhatt almost 6 yearstry this app.use(cors()); var port = process.env.PORT || 9090; app.get('/', function(req, res) { res.send('Hello!'); }); app.listen(port); console.log('Server started.');
-
Chiien almost 6 yearsWell, try with * =), and use that app.use(function (req, res, next) { res.setHeader('Access-Control-Allow-Origin', 'localhost:8888'); res.setHeader('Access-Control-Allow-Methods', 'GET, POST, OPTIONS, PUT, PATCH, DELETE'); res.setHeader('Access-Control-Allow-Headers', 'X-Requested-With,content-type'); res.setHeader('Access-Control-Allow-Credentials', true); next(); });
-
Ronnie over 5 yearsHere is a better solution though: github.com/jeromeetienne/AR.js/issues/…