Angular 7 Test: NullInjectorError: No provider for ActivatedRoute
Solution 1
Add the following import
imports: [
RouterModule.forRoot([]),
...
],
Solution 2
You have to import RouterTestingModule
import { RouterTestingModule } from "@angular/router/testing";
imports: [
...
RouterTestingModule
...
]
Solution 3
I had this error for some time as well while testing, and none of these answers really helped me. What fixed it for me was importing both the RouterTestingModule and the HttpClientTestingModule.
So essentially it would look like this in your whatever.component.spec.ts file.
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [ProductComponent],
imports: [RouterTestingModule, HttpClientTestingModule],
}).compileComponents();
}));
You can get the imports from
import { RouterTestingModule } from "@angular/router/testing";
import { HttpClientTestingModule } from "@angular/common/http/testing";
I dont know if this is the best solution, but this worked for me.
Solution 4
This issue can be fixed as follows:
In your corresponding spec.ts file import RouterTestingModule
import { RouterTestingModule } from '@angular/router/testing';
In the same file add RouterTestingModule as one of the imports
beforeEach(() => { TestBed.configureTestingModule({ imports: [RouterTestingModule], providers: [Service] }); });
Solution 5
Example of a simple test using the service and ActivatedRoute! Good luck!
import { async, ComponentFixture, TestBed } from '@angular/core/testing';
import { RouterTestingModule } from '@angular/router/testing';
import { HttpClientModule } from '@angular/common/http';
import { MyComponent } from './my.component';
import { ActivatedRoute } from '@angular/router';
import { MyService } from '../../core/services/my.service';
describe('MyComponent class test', () => {
let component: MyComponent;
let fixture: ComponentFixture<MyComponent>;
let teste: MyComponent;
let route: ActivatedRoute;
let myService: MyService;
beforeEach(async(() => {
teste = new MyComponent(route, myService);
TestBed.configureTestingModule({
declarations: [ MyComponent ],
imports: [
RouterTestingModule,
HttpClientModule
],
providers: [MyService]
})
.compileComponents();
}));
beforeEach(() => {
fixture = TestBed.createComponent(MyComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('Checks if the class was created', () => {
expect(component).toBeTruthy();
});
});
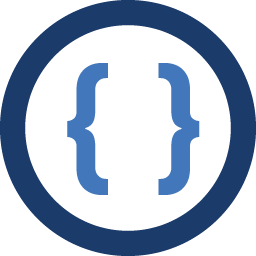
Admin
Updated on July 16, 2022Comments
-
Admin almost 2 years
Hi have some error with testing my App made with Angular 7. I do not have much experience in angular, so I would need your help+
Error: StaticInjectorError(DynamicTestModule)[BeerDetailsComponent -> ActivatedRoute]: StaticInjectorError(Platform: core)[BeerDetailsComponent -> ActivatedRoute]: NullInjectorError: No provider for ActivatedRoute!
the testing code is like this:
import { async, ComponentFixture, TestBed, inject } from '@angular/core/testing'; import { BeerDetailsComponent } from './beer-details.component'; import { HttpClientTestingModule, HttpTestingController } from '@angular/common/http/testing'; describe('BeerDetailsComponent', () => { let component: BeerDetailsComponent; let fixture: ComponentFixture<BeerDetailsComponent>; beforeEach(async(() => { TestBed.configureTestingModule({ imports: [HttpClientTestingModule], declarations: [ BeerDetailsComponent ] }) .compileComponents(); })); beforeEach(() => { fixture = TestBed.createComponent(BeerDetailsComponent); component = fixture.componentInstance; fixture.detectChanges(); }); it('should create', inject( [HttpTestingController], () => { expect(component).toBeTruthy(); } ) ) });
I really can't find any solution.
Daniele
-
Admin over 5 yearswhere should i import RouterModule.forRoot([]),?
-
Sachila Ranawaka over 5 yearsIn the module
import { RouterModule } from '@angular/router';
-
Sachila Ranawaka over 5 years:) make sure the check as answer if this helped.
-
Arjun Panicker about 4 yearsRouterModule should not be included in the test files
-
cagcak almost 4 yearsthats not a proper solution, by this you need to define all roter module provider params, APP_BASE_HREF token etc. Use instead RouterTestingModule with no router provider declarations
-
Ross Brasseaux almost 4 yearsWhen I do this, I start getting the error
NullInjectorError: No provider for ActivatedRoute!
-
RaulDanielPopa almost 4 yearsDid you imported this module into the spec file?
-
Ross Brasseaux almost 4 yearsI believe the solution was to add a provider for the route, using an anonymous object containing the properties accessed by the test.
-
JSON derulo over 3 yearsAre you using
teste
anywhere? for what is it needed? -
Cherma Ramalho over 3 yearsI use everywhere I work, a way to ensure that the application keeps working after some change without breaking my code.
-
NobodySomewhere over 3 yearsWould be a good answer if you stated where the import should be added
-
Jifri Valanchery over 3 years@daniele-caputo, make sure that you have accepted the currect answer. other answers with import: [RouterTestingModule] resolved my error.
-
steve.lippert about 3 yearsThe correct answer is provided by @prathvi-shetty below.
-
Alfredo A. over 2 yearsThis should be the accepted answer
-
Mike Poole almost 2 yearsIf you are coming to this in 2022 then
RouterTestingModule
now lives in@angular/common/http/testing