Angular 8 Observable.interval changed?
12,072
Solution 1
Can you try something like this:
import { interval } from 'rxjs';
interval(1000).subscribe(x => {
// something
});
Solution 2
Use timer
if you want to wait n seconds before the first run or run it immediately and then every n seconds.
Wait 3 seconds and then run every second:
import { timer } from 'rxjs';
timer(3000, 1000).subscribe(x => {
// something
});
Run immediately and after that every 1 second (I don't know if this is the most elegant way to do it, but it's much simpler than other solutions I've seen, and it works):
import { timer } from 'rxjs';
timer(0, 1000).subscribe(x => {
// something
});
Solution 3
*// Use RxJS v6+ and emit value in sequence every 1 second
import { interval } from 'rxjs';
const source = interval(1000);
const subscribe = source.subscribe(val => console.log(val));
//output: 0,1,2,3,4,5....
Solution 4
you can use interval like below. It works in angular12 also.
import { interval } from 'rxjs';
export class ObservableComponent implements OnInit, OnDestroy {
intervalSubscription: Subscription;
source = interval(1000);
constructor() {}
ngOnInit(): void {
/*every 1second it emits value 0,1,2...
if we move to other component(destroy the component) it won't stop
incrementing and another interval created along with the old
interval. Untill we unsubscribed it it
won't stop the incrementing process
*/
this.intervalSubscription = this.source.subscribe(val =>
console.log(val));
}
ngOnDestroy() {
//when you move to another component, now the interval will be
//unsubscribed.
this.intervalSubscription.unsubscribe();
}
}
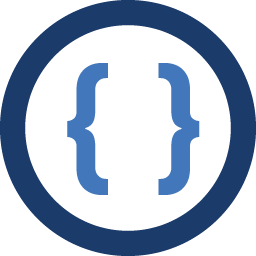
Author by
Admin
Updated on June 27, 2022Comments
-
Admin almost 2 years
I am trying to use Observable.interval on angular 8 and it doesn't seem to like it.
First I import rxjs:
import { Observable } from 'rxjs';
Then the code:
Observable.interval(1000).subscribe(x => { // something });
What is the angular 8 syntax?