Angular and text-mask: Regex to validate an input without special characters
Solution 1
This text-mask is not a regular expression. Documentation says that
mask
is an array or a function that defines how the user input is going to be masked. Each element in the array has to be either a string or a regular expression. Each string is a fixed character in the mask and each regular expression is a placeholder that accepts user input.
Your mask is an array containing one character. This means that it will accept only one character that matches /^[a-zA-Z]+$/
.
If you want multiple characters, you need an array of regular expressions for each of them, like this:
public mask = [/[a-zA-Z]/, /[a-zA-Z]/, /[a-zA-Z]/];
This mask will accept three letters.
Solution 2
I know it happened a few months ago but I found a way to do it.
You can create a function which receives as a parameter the raw value and returns a regexp array.
So, you just basically receive the raw value, count the lenght and you push the mask to the array.
I did it like this:
<input [textMask]="{mask: nameMask, guide: false}" [(ngModel)]="myModel" type="text"/>
nameMask(rawValue: string): RegExp[] {
const mask = /[A-Za-z]/;
const strLength = String(rawValue).length;
const nameMask: RegExp[] = [];
for (let i = 0; i <= strLength; i++) {
nameMask.push(mask);
}
return nameMask;
}
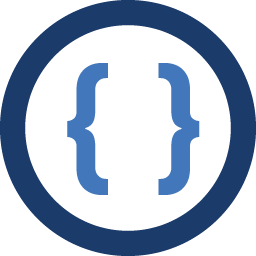
Admin
Updated on June 24, 2022Comments
-
Admin about 2 years
I have angular 5 with "angular2-text-mask": "^8.0.4" https://github.com/text-mask/text-mask/tree/master/angular2
What I'm trying to do is preventing users entering special characters, except for -, _, ', which should be allowed for a
name type="text"
input.This works for phone numbers:
<input [textMask]="{mask: mask}" [(ngModel)]="myModel" type="text"/> public myModel = '' public mask = ['(', /[1-9]/, /\d/, /\d/, ')', ' ', /\d/, /\d/, /\d/, '-', /\d/, /\d/, /\d/, /\d/]
But when I try
public mask = [/^[a-zA-Z]+$/];
which is supposed to accept multiple letters, however I can only write one. What's wrong? I like to be able to enter unlimited letters, digits and -, _, 'I tested my mask at https://regex101.com/
Any help is appreciated.
-
My Stack Overfloweth about 6 yearsunfortunate that the feature isn't supported, but thank you for confirming it.
-
Villarrealized over 4 yearsThanks, this helped! For others looking, you can reduce this down to a one-liner:
const nameMask = (rawValue => [...rawValue].map(() => /[A-Za-z]/));