Angular google maps (AGM) autocomplete
13,537
We can find some details using google maps api, follow the steps.
step 1: Import MapsAPILoader
to your component.
import { MapsAPILoader } from '@agm/core';
@ViewChild('search') searchElementRef: ElementRef;
step 2: Implement findAdress()
method and call it in your ngAfterViewInit()
method.
findAdress(){
this.mapsAPILoader.load().then(() => {
let autocomplete = new google.maps.places.Autocomplete(this.searchElementRef.nativeElement);
autocomplete.addListener("place_changed", () => {
this.ngZone.run(() => {
// some details
let place: google.maps.places.PlaceResult = autocomplete.getPlace();
this.address = place.formatted_address;
this.web_site = place.website;
this.name = place.name;
this.zip_code = place.address_components[place.address_components.length - 1].long_name;
//set latitude, longitude and zoom
this.latitude = place.geometry.location.lat();
this.longitude = place.geometry.location.lng();
this.zoom = 12;
});
});
});
}
step 3: Make some changes in your html file.
<input #search autocorrect="off" autocapitalize="off" spellcheck="off" type="text" class="form-control">
step 4: don't forget to add places library in your AgmCoreModule
configuration.
import { AgmCoreModule } from '@agm/core';
imports: [
AgmCoreModule.forRoot({
apiKey: 'your_key',
libraries: ["places"]
})
]
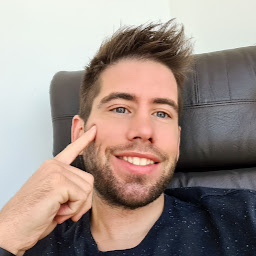
Author by
Matej Mejak
Updated on June 07, 2022Comments
-
Matej Mejak almost 2 years
I'm using angular google maps (AGM) to find addresses. Now I would like that when a person choose and address it fills the inputs. Where can I find the single address element names like street, str. number, postal code, etc. to bound the data to the input field?
-
henrisycip over 6 yearscould you post your sample in a [plunker](plnkr.co) or demo environment, so that we can see your progress so far while using agm?
-
-
Murphy4 over 5 yearsTHANK YOU! As of Angular 6+ my tests suddenly started failing because I forgot to include
libraries: ["places"]
in my spec.ts imports -
Adnan over 4 yearsWhere new google.maps.places.Autocomplete comes from?
-
Liam Hogan over 3 years$ npm install @types/googlemaps --save-dev stackoverflow.com/questions/36064697/…